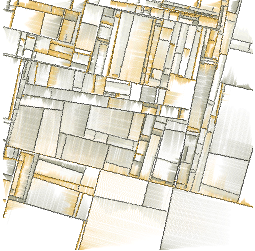
Demos below!
As a sort-of reverse birthday present I’ve decided to release one of my largest projects, in recent memory. This is the project that I’ve been alluding to for quite some time now:
I’ve ported the Processing visualization language to JavaScript, using the Canvas element.
I’ve been working on this project, off-and-on now, for the past 7 months – it’s been a fun, and quite rewarding, challenge. The full scope of the project can be broken down into two portions:
The Processing Language
The first portion of the project was writing a parser to dynamically convert code written in the Processing language, to JavaScript. This involves a lot of gnarly regular expressions chewing up the code, spitting it out in a format that the browser understands.
It works “fairly well” (in that it’s able to handle anything that the processing.org web site throws at it) but I’m sure its total scope is limited (until a proper parser is involved). I felt bad about tackling this using regular expressions until I found out that the original Processing code base did it in the same manner (they now use a real parser, naturally).
The language includes a number of interesting aspects, many of which are covered in the basic demos. Here’s a brief selection of language features that are handled:
- Types and type casting – Type information is generally discarded, but becomes important in variable declaration and in casting (which is generally handled well).
- Classes – The full class system is supported (can be instantiated, etc. just fine).
- Method overloading and multiple constructors – Within classes you can have multiple method (or constructor) definitions – with the appropriate methods being called, based upon their signature length.
- Inheritance – Even classical-style inheritance is supported.
Note: There’s one feature of Processing that’s pretty much impossible to support: variable name overloading. In Processing you can have variables and functions that have the same name (e.g. float size = 0; float size(){}
). In order to support this there would have to be considerable overhead – and it’s generally not a good practice to begin with.
If you’re curious as to what the language looks like, here’s a basic example of inheritance:
class Spin { float x, y, speed; float angle = 0.0; Spin(float xpos, float ypos, float s) { x = xpos; y = ypos; speed = s; } void update() { angle += speed; } } class SpinArm extends Spin { SpinArm(float x, float y, float s) { super(x, y, s); } void display() { strokeWeight(1); stroke(0); pushMatrix(); translate(x, y); angle += speed; rotate(angle); line(0, 0, 66, 0); popMatrix(); } }
The Processing API
The second portion of the project is the full 2d Processing API. This includes all sorts of different methods:
- Shapes drawing
- Canvas manipulation
- Pixel utilities
- Image drawing
- Math functions
- Keyboard and mouse access
- Objects (point, arrays, random number generators)
- Color manipulation
- Font selection and text drawing
- Buffers
Most of these are demonstrated in the basic demos.
There’s pretty-good coverage of the Processing API: there’s sure to be many gaps, but most of what I can throw at it works.
Download
The full source code is contained within a single file. It comes in at about 5000 lines, compresses down to less than 10kb.
How to Use
The API is quite simple – there’s a single method “Processing”. If you wish to only use the Processing API (not the language) you can interact with it like so:
var p = Processing(CanvasElement); p.size(100, 100); p.background(0); p.fill(255); p.ellipse(50, 50, 50, 50);
If you wish to have the full power of the language, as well, you would pass in your Processing code as the second argument.
Processing(CanvasElement, "size(100, 100); background(0);" + "fill(255); ellipse(50, 50, 50, 50);");
That’s really all there is to it. Information on the full Processing API can be found on the Processing.org web site.
Important: Browser Support
Before we get into the demos I want to outline what some of my goal was for this project. First, and foremost, I wanted to try and get the best Canvas-based demos out of a browser, as possible. This meant that I had to shoot directly for the latest, and greatest, browsers. I needed good Canvas API support and, importantly, I needed speed.
Because of this, for this first release, I’ve specifically targeted Firefox 3, the latest WebKit Nightly, and Opera 9.5. In other words: beta browsers.
A large part of the code base is sure to work in “older” browsers (Firefox 2, Safari 3, Opera 9, and IE with excanvas) – but that wasn’t my target platform. I wanted something that would be capable of pushing the edge of what a browser is able to render – giving them something to strive for in their upcoming releases.
There were a couple of stumbling blocks that the above browsers hit:
- Image loading – Currently, only Firefox 3, Opera 9.5, and Safari 3.1 handle this reliably.
- Pixel processing – Loading pixel data from images, manipulating them, and putting them back on the canvas. Only Firefox 3, Opera 9.5, and WebKit Nightlies can handle this sufficiently.
- Font loading and text rendering – The specification for this is still in the works, currently only Firefox 3 has support for this.
Thus, anything outside of the above (images, pixel processing, and text) should work “ok” everywhere.
Demos
NOTE: I highly recommend that you use the latest Firefox 3 beta to view the demos. Most will work in the latest WebKit Nightly and a majority will work in Opera 9.5, but all will work in Firefox 3.
Note again: A lot of these demos will peg your CPU. As I mentioned above, I’m trying to squeeze the most out of the browser, as possible – be ready for it!
What would the release be without a ton of demos? In development I worked in a backwards manner. Instead of building the API up from the ground – I worked from the top, down, implementing enough of the API to get individual demos working. The result of this is two-fold: 1) It made for a very rewarding development process – and guaranteed working demos and 2) It means that there are still portions of the Processing API left unimplemented.
Regardless – enough of the API has been implemented to allow all of the demos, available on processing.org, to work as best as possible.
Here’s the full break-down of demos that are available:
- 91 basic demos. – All of the demos were written by Casey Reas and Ben Fry unless otherwise stated.
- 51 larger, topical, demos. – All of the demos were written by Casey Reas and Ben Fry unless otherwise stated.
- 4 custom “in the wild” demos.
I’ve gone through and cherry-picked a bunch that I really liked – in case you’re interested in seeing some really interesting ones. Wherever possible I specify what browsers the demos work in (if none is specified, then it should work in all).
Custom Built/”Found In the Wild” Demos
Basic Demos (91 Total)
All of the following demos were written by Casey Reas and Ben Fry unless otherwise stated.
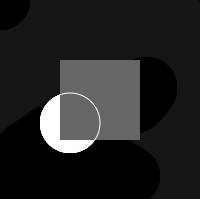
Using buffers to draw multiple, simultaneous, canvases. (Firefox 3, Opera 9.5)
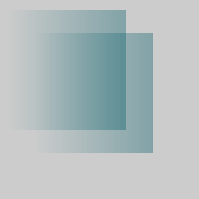
Dynamically drawing multiple, dynamically-generated, transparent, images. (Firefox, Opera 9.5)
Topical Demos (51 Total)
All of the following demos were written by Casey Reas and Ben Fry unless otherwise stated.

Adjusting brightness on an image. (Firefox, Opera 9.5)

Scanning the pixels of an image. (Firefox, Opera 9.5)
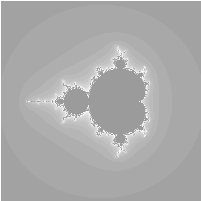
Mandelbrot Fractal (Firefox, Opera 9.5)
I’m quite curious to see what people construct with this new API. I think it holds a lot of potential and I’m excited to see what comes of it! Enjoy!
Remember: You should be using Firefox 3, a WebKit Nightly (Safari 3.1 is missing some features), or Opera 9.5 – the above demos generally work best in those browsers.
Jeff Eaton (May 8, 2008 at 10:04 pm)
I’ll just take a moment to go slack-jawed. Holy crap, man. That’s awesome.
Aaron (May 8, 2008 at 10:10 pm)
?!!!?!?!?!?!! ?!! … !!!
alex guzman (May 8, 2008 at 10:11 pm)
this is insane!
AndrewT (May 8, 2008 at 10:12 pm)
What Jeff said….
Jason Persampieri (May 8, 2008 at 10:15 pm)
Wow. Holy crap.
Rich Manalang (May 8, 2008 at 10:17 pm)
Unbelievable. Great work, dude. I can’t wait to try this out.
PNuin (May 8, 2008 at 10:19 pm)
Looks really nice, will definitely try it. Cheers
Tom Dyer (May 8, 2008 at 10:20 pm)
Great, Processing without Java.
Eduardo Lundgren (May 8, 2008 at 10:21 pm)
It sounds good.
Congratulations for the initiative in building a parser for this powerful open source programming language.
What we have to do is help and cheer this amazing project to let the Processing.js support each more demos – and make it a strongly parser.
It will be very important for the future of images, animation, and interactions on a browser.
Abi (May 8, 2008 at 10:22 pm)
I just started trying out processing about a month ago. Now with javascript, its going to be totally awesome. Great work!
Blake (May 8, 2008 at 10:24 pm)
Man, this is some awesome work, can’t wait to find an excuse to use it.
jessamyn (May 8, 2008 at 10:30 pm)
Happy Birthday indeed!
rektide (May 8, 2008 at 10:35 pm)
birthday #0
Scott Johnson (May 8, 2008 at 10:37 pm)
Wow, this is huge!
Rob L. (May 8, 2008 at 10:44 pm)
Yowza, this looks cool; can’t wait to dig in. Happy birthday, John, and thanks.
Mauro (May 8, 2008 at 10:54 pm)
this is just freaking insane.
Coto (May 8, 2008 at 11:11 pm)
Fantástico John… Fantástico
Oliver (May 8, 2008 at 11:12 pm)
Very cool demo :D
It looks like a number of the tests are failing in webkit because they are relying on non-standard gecko-isms rather than webkit bugs. The biggest issue appears to be that a number of the tests use a generic object for ImageData rather than an actual ImageData object created with Canvas.createImageData. Using a generic object is not safe behaviour as it fails to account for the canvas being resolution independent — another issue is that it means that future optimisations to the ImageData representation used by engines will not be made available to you :-(
A simple place where this occurs is in updatePixels for the mandelbrot demo, you use
var pixels = {};
var data = pixels.data = [];
pixels.width = p.width;
pixels.height = p.height;
Where the “correct” approach would be
var pixels = .createImageData(p.width, p.height);
This is still ignoring the problem that the number of CSS pixels you request may not necessarily match the number of physical pixels you get back, but should work in Opera and WebKit nightlies.
Einar Vollset (May 8, 2008 at 11:23 pm)
I’m speechless. Great work.
Iraê (May 8, 2008 at 11:25 pm)
Impressive! I can’t even think for an apropriate way to complement you is this awesome great project!!!
Hope to see great stuff that came from that on the JSNinja book! =D
Avi Flax (May 8, 2008 at 11:28 pm)
Wow.
Lincoln (May 8, 2008 at 11:28 pm)
I nominate you for the position of God. Unbelievable.
manfre (May 8, 2008 at 11:38 pm)
Very nice. I wonder how long until some one creates a javascript game that is on par with flash.
Daniel Raffel (May 8, 2008 at 11:39 pm)
Holy cow, brilliant work John!
Scott Lawrence (May 8, 2008 at 11:39 pm)
Damn, man. That’s really impressive. Nice job!
-s (fellow CSH’er)
P.J. Onori (May 8, 2008 at 11:45 pm)
I am simply blown away. This is amazing.
Kamran (May 8, 2008 at 11:54 pm)
Wow,this is incredible, really impressive work, changes the way I look at javascript.
david gurba (May 9, 2008 at 12:08 am)
Ragel parser builder :) … just code a new Javascript backend :/ Then the Processing.org people and the Processing.js people could stay in sync.
Eric D (May 9, 2008 at 12:29 am)
Simply amazing, way to push the envelope. and 10k! Thank you, sir.
Ara Pehlivanian (May 9, 2008 at 12:31 am)
Extremely impressive dude… you totally rock. You _are_ fully human right? Man.
h3 (May 9, 2008 at 12:36 am)
Fortunately you’re always there to impress us and pull back the limits of JavaScript :)
Thank you and kudos.
Andrew (May 9, 2008 at 12:41 am)
Animator is my favourite I think http://ejohn.org/apps/processing.js/examples/topics/animator.html
Kevin Weibell (May 9, 2008 at 12:58 am)
Wooohoooo, this is so awesome!! Well done, John!
Johann (May 9, 2008 at 1:09 am)
I’m speechless.
Sean (May 9, 2008 at 1:35 am)
Mind Blowing! Great Work
beppu (May 9, 2008 at 1:35 am)
You have outdone yourself.
daaku (May 9, 2008 at 1:36 am)
mind boggling!
gally (May 9, 2008 at 1:52 am)
WOW ! Congratulation, this is great.
niko (May 9, 2008 at 1:58 am)
erm… nothing to say that hasn’t been said before… but… wow^10!
Mark (May 9, 2008 at 2:10 am)
Stunning.
Colin Ramsay (May 9, 2008 at 2:41 am)
Amazing work.
jive (May 9, 2008 at 2:50 am)
wow. so big and pretty! I am really impressed. I’ve never seen javascript animate so smoothly, as flash. This will really take off.
Ozh (May 9, 2008 at 2:55 am)
Holy cow.
(flash is dead?)
qq (May 9, 2008 at 2:57 am)
Finally, flash animation has real rival from ajax world . Great!
doom (May 9, 2008 at 3:02 am)
hey john .. that’s cool …
someday .. we going to have direct3D ajax so that we can make a 3D game :D
– JQuery direct3D / OpenGL Plugins … :D
Sebastian Redl (May 9, 2008 at 3:10 am)
This is truly amazing. And I agree with Andrew, animator is fascinating. And addicting.
On a side note, all three links in your demo listing are broken. They all go to apps/processing/ instead of apps/processing.js/ and the third one ends in csutom or something like that instead of custom.
Luke (May 9, 2008 at 3:29 am)
Great work John – is this what you demoed at @media Ajax last year?
Happy birthday by the way!
Jauhari (May 9, 2008 at 3:31 am)
Just Perfect ;)
Adam (May 9, 2008 at 3:43 am)
Wa wa we wah, wow this is fantastic, amazing work.
J.W (May 9, 2008 at 3:44 am)
Happy birthday John! and congratulations! this is really great project
Olle Jonsson (May 9, 2008 at 3:53 am)
We. Are. In. Awe.
(Just began reading that Visualizing Data book, 2 days ago. How is your timing this great?)
Nilesh (May 9, 2008 at 4:05 am)
John – you’re a robot from the future.
Absolutely amazing work.
Josue R. (May 9, 2008 at 4:08 am)
Happy Birthday. I wish my bdays could be this awesome too!
bonersawarded (May 9, 2008 at 4:12 am)
Maybe I’m missing the point here… but hasn’t everything in these demos been possible in flash since pretty much the first version? and isn’t flash scripting pretty much as terse and concise as the kooky drawing language that this library hacks into javascript? just a thought…
Charles Phillips (May 9, 2008 at 4:13 am)
Incredible, John. Are you a demoscene fan (see scene.org)? I noticed you call these all ‘demos’… This reminds me of demo effects, but written in canvas. I’ve seen this in the browser with Flash and Java, but of course you had to bring it to JS. I’m sure by now you must be familiar with p01 (po1.org) …
Always great to see languages pushed to the edge. This will be a long-lasting effect on JavaScript and vector graphics in the browser. Can’t wait to see where it goes from here!
Patrick (May 9, 2008 at 4:22 am)
OMG
i never thought that such things could be done with JS!
czert (May 9, 2008 at 4:22 am)
Incredible work.
There really is something about the whole “large and pretty” thing .)
Kristof Neirynck (May 9, 2008 at 4:22 am)
Wonderful.
I wonder weather this could be ported to ActionScript 3.
Alistair (May 9, 2008 at 4:43 am)
If they ask why then they don’t understand. Some things are just cool things to do. Kudos to you.
Vaska (May 9, 2008 at 4:46 am)
John must live in the future and be reporting back to us. Amazing!
Dan W (May 9, 2008 at 4:47 am)
Happy Birthday!
Jason (May 9, 2008 at 4:53 am)
Well done, congratulations John.
Just the other day when you posted about Processing I kinda tied the two together (sneak peak). Those who have asked if this is possible in Flash, well yes of course it is. However this is open, un-encapsulated and on the whole a far superior angle of attack.
I look forward to seeing you @media later this month
Happy birthday dude ;)
P.S. WOW!
lrbabe (May 9, 2008 at 4:58 am)
Happy birthday and congratulations, you are my hero.
Mauro De Giorgi (May 9, 2008 at 5:25 am)
OMG… this is incredible…
Sandro (May 9, 2008 at 5:30 am)
o_O O_O O_o
WOA !! That’s amazing !
Thanks !
Sandro
Asbjørn Ulsberg (May 9, 2008 at 5:32 am)
Happy birthday and thank you so much for this amazing present to the web community! Phenomenal work, John! Truly incredible stuff. Looking forward to seeing better support and cross-browser compatibility for
<canvas />
in the following months.The really big disappointment of course, is — as always — Internet Explorer. I wonder when that big heap of stinking, bloated and non-conformant piece of C++ code called a browser will start supporting anything but Microsoft’s own proprietary crap, including the
canvas
element and SVG.Speaking of SVG, could that be an alternative renderer for
Processing
?jmdesp (May 9, 2008 at 5:37 am)
Is it me or is the Animator exemple actually faster in the javascript version ?
Marc Diethelm (May 9, 2008 at 5:49 am)
Hi John, hope you had a nice birthday!
# 51 larger, topical, demos.
# 4 custom “in the wild” demos.
Those links result in a 404.
Nice work!
Roshan Bhattarai (May 9, 2008 at 6:10 am)
wow……..thanks for this……
Dot. (May 9, 2008 at 6:10 am)
Happy birthday John!
Awesome work!
Though the links for
* 91 basic demos.
* 51 larger, topical, demos.
* 4 custom “in the wild” demos.
are wrong, the base url should be /apps/processing.js/examples/ instead of /apps/processing/examples/ and you misspelled “csutom”.
Hadrien (May 9, 2008 at 6:39 am)
Happy birthday,
It just totally rocks !
John Griffiths (May 9, 2008 at 6:39 am)
excellent work, the glass door effect is really nice. can so see a use for the dynamic bar chart.
well done!
Daniel Shiffman (May 9, 2008 at 7:10 am)
Totally awesome
Hamish M (May 9, 2008 at 7:19 am)
Really amazing work, John. I never expected this.
Thanks for all your hard work, this couldn’t have been easy!
David Goodwin (May 9, 2008 at 7:31 am)
Great demos – it looks very exciting .. now we’ll just have to wait a year or two before we can deploy stuff based on it
p3 (May 9, 2008 at 7:32 am)
Very nice. I think using this with xul runner gives quite many interesting possibilities, now I only need some time to develop something.
By the way. Most of the demos worked pretty well in Firefox 2. I was expecting most of them to fail and only few to work, but instead it was the other way around.
Doc (May 9, 2008 at 7:47 am)
oh my – i have to go change i got so excited.
really really cool stuff.
dani (May 9, 2008 at 7:56 am)
incredible!!
dimitre (May 9, 2008 at 8:00 am)
JOHN this is fantastic! this will change some serious things out there
Zach Leatherman (May 9, 2008 at 8:15 am)
But… I thought the applet was making a comeback?
I guess not.
Lo J (May 9, 2008 at 8:22 am)
I LOVE YOU john, do you want to marry me ? your brain is so sexy.
mikx (May 9, 2008 at 8:31 am)
Amazing!
Laurian Gridinoc (May 9, 2008 at 8:34 am)
Bliss! I was switching to an Adobe AIR UI and crying after Processing, now I can do it with canvas in html in AIR.
Ivan (May 9, 2008 at 8:41 am)
Impressive. These are the fun projects you do in your spare time? I like to play around with JQuery menus plugins! hah.
It’s sorta timely that I just finally bought visualizing data by Ben Fry literally a week ago.
Jonathan Christopher (May 9, 2008 at 8:48 am)
I know you’ve heard it umpteen times, but this is ridiculously awesome. I’m flat out amazed.
Jeremy Zawodny (May 9, 2008 at 9:10 am)
Uhm… Kick Ass!
Theun de Bruijn (May 9, 2008 at 9:15 am)
Awesome.
Finally a way to bring Processing to the masses.
Big thumbs up and heaps of thanks ;)
Mason Wendell (May 9, 2008 at 9:16 am)
Wow, John. This is incredible. Can’t wait to play with it. Happy Birthday.
_why (May 9, 2008 at 9:46 am)
Golly juggle hats! This is totally sensational work, kid. Normally, I would feel bad for Processing people (since this will clearly eclipse their whole distro) but you’ve done them a favor by circumventing the applet. So, everyone wins I guess.
James (May 9, 2008 at 9:54 am)
Hi John,
This is great stuff. Really cool.
I’ve messed about with Processing a few years ago, but performance always put me off – as you say, it pegs your CPU.
As I read your post, I was wondering if you going to do the translation from p5 to js in the browser or before hand – as you know p5 has an offline step which takes p5 source, translates it to Java source then compiles with jikes.
More recently, I’ve found GWT; which contains an optimizing compiler for Java source to js.
Unfortunately, your comment about p5’s performance is the killer for p5, for me anyhow. For it to “break out” for wider usage – including this project – it needs to be sort the busy-waiting loop out. I know that it’s optimized (or it seems to be) for FPS rather than being kind to the CPU, so it’s not something that is easily fixed.
In which case, I’d be really interested to look at the canvas implementation of the API (not done that yet); perhaps it could be wrapped in to a GWT implementation, so it could be used from java!?
Thanks
Ned Schwartz (May 9, 2008 at 10:20 am)
Wow!
Just amazing.
Neelesh (May 9, 2008 at 10:26 am)
This rocks! Awesome!
tzMedia (May 9, 2008 at 10:40 am)
Nice John… you’ve just renewed your status as “über Geek 2008-09”.
Awesome stuff, looks like you’ve put in a great dill-yio of work here.
thanks!
Leandro (May 9, 2008 at 10:43 am)
Impressive!
You’re the man!
Tom (May 9, 2008 at 10:54 am)
I still want to see the abstract API over canvas, Flash, and Silverlight so that it just works. Maybe excanvas is the place to do that, making it use Flash or Silverlight if available rather than VML.
Carson (May 9, 2008 at 11:13 am)
My mind. It is blown.
Jarrod (May 9, 2008 at 11:13 am)
Jaw-droppingly amazing. Happy birthday.
Josh (May 9, 2008 at 11:14 am)
Awesome, and insane, as others have posted. Very cool work.
I wonder though if there isn’t a way let processing be processing, and instead write a nice JS/Java bridge to interact with it on a page. Isn’t Java always going to be faster? Am I being naive in thinking that it’s pretty widely installed on people’s machines?
Wade Harrell (May 9, 2008 at 11:26 am)
this makes me want to get back in to PhotoShop scripting like i was doing way back in ’00 while working on videos like http://traunic.com/img/video/ndfh.mov
stuff that is animated saves out a file for each frame then used After Effects to string it all together and mix. i can just imagine the watercolor piece at 1080p and a 42″+, would be amazing!
great work!!!
David Golightly (May 9, 2008 at 11:30 am)
John Resig, you are my hero.
The Processing parser/interpreter is interesting for directly executing existing processing code, but I’m mostly interested in the JS API – I’m going to be doing all my Processing in JS from now on, rather than their older version of Java. Thank you, thank you, thank you!
Neal (May 9, 2008 at 11:52 am)
Yikes, incredible work John. Thanks.
misha (May 9, 2008 at 12:02 pm)
awesome
Dale Harvey (May 9, 2008 at 12:17 pm)
This is really good stuff, it looks like something that could really push canvas adoption.
Noticed one minor issue (ff3) is that the mouse position doesnt take into account the page scroll, ie on the snake demo if you scroll the page slightly the sname will follow however much you have scrolled above the mouse.
Ill certainly be playing around with this though, thanks
Anon Dude (May 9, 2008 at 12:24 pm)
Congrats and great work!
The demos look very similar to IE canvas demos. The HTML canvas is plagued by the lack of a drawString() type of function. All graphics but no text. A simple chart with header/footers for values is not doable in canvas. Is there a method for drawing text in Processing?
kestasjk (May 9, 2008 at 12:25 pm)
Happy birthday, you rule. The Java requirement always held Processing back, this is going to make Processing more widely used than ever. I can’t wait for a good excuse to make use of this myself
Steve (May 9, 2008 at 12:26 pm)
It would be nice to a big image zooming/panning tool implemented in this.
Daniel Bigham (May 9, 2008 at 12:28 pm)
Marvelous! Your work really tickles me! First of all, this is the first time I’ve seen the processing visualization language, and it looks really interesting. Second of all, I’m really impressed that you’re rendering such nice, interactive images with Javascript.
Well done! I’ll have to spend some time examining PVL. I’m also interested in how one might be able to tightly integrate PVL or something PVL-like into a fuller programming language. It’s always been one of my beefs, and I think for lots of kids, that it’s not easier to draw pretty pictures using programming languages.
Hedger Wang (May 9, 2008 at 12:32 pm)
This is definitely the best DHTML/Canvas project and the most creative and robust work I’ve ever seen.
Excellent job, John!!!
Bruno (May 9, 2008 at 12:36 pm)
It seems flash will only be used to display videos and building flex applications in a short future. If at all. =P
EML (May 9, 2008 at 12:57 pm)
“One Language To Rule Them All, One Language To Find Them, One Language To Bring Them All And In The Browser Bind Them!”
Have to stop typing before I start coursing. #$%@? awesome.
Dr. Mohan (May 9, 2008 at 1:16 pm)
Amazing!
Tim Hurt (May 9, 2008 at 1:18 pm)
Bravo. I dig it.
Ray Cromwell (May 9, 2008 at 1:54 pm)
Awesome job! I’ve been mulling over doing something like this port for some time, since we’re working on building a easy to use end user visualization platform, but CPU issues put me off.
@James,
With GWT, you can avoid runtime parsing and use GWT’s deferred binding mechanism to “compile” the processing language at compile time to JS, you could also generate ActionScript which might help with feature issues related to Canvas. If you’re interested in using a JS/Java canvas API that works fast across browsers, see http://timepedia.org/chronoscope/demo/?_force_flash , which contains a GWT Canvas implementation on-top of either the CANVAS tag, or Flash. It is exportable to JS as a minified/packed library, so doesn’t require GWT usage if you don’t want GWT.
-Ray
steve cooley (May 9, 2008 at 2:24 pm)
This is off the charts awesome. I look forward to seeing how many of my sketches port over well!
Credit where credit is due in one of your examples. The example named “artistic water-color style drawing” is either entirely written or enormously inspired by Jared Tarbell.
http://www.complexification.net/
Brett (May 9, 2008 at 2:35 pm)
Hey John,
I started work on a project, a while back, but getting people interested in it is quite problematic. I’ve always thought Javascript was a great language for prototyping and developing games. The problem is that it requires (as you noted) a lot of work.
I started a game engine in Javascript, but haven’t had a lot of time to work on it lately. It uses Dean Edwards’ “Base” class to simplify object inheritance. I also use jQuery and some other libs to simplify things a bit.
There’s an example of an Asteroids clone that works in FF2 and FF3, right now, with support for developing other games. I want to create some more examples, but alas, time is not a friend as I’m sure you know.
The concept was to create an engine that allows a developer to “plug-in” any of many renderers. Right now, the most complete is the Canvas render context. I had also hoped to build an HTML render context, an SVG one, and potentially a VML render context. Additionally, it’s using a concept that a few guys, and myself, developed for the componentization of game objects.
The lib is still early in development, but could use some eyes on it and a team for development. Who knows… maybe someday it could be another useful JS app. =)
– Brett
Keith (May 9, 2008 at 2:37 pm)
Happy birthday John :) Rockin library. Keep it up!
psyjoniz (May 9, 2008 at 2:45 pm)
great work. can’t wait to find a reason to utilize it.
Ray Cromwell (May 9, 2008 at 3:04 pm)
Awesome job! I’ve been mulling over doing something like this port for some time, since we’re working on building a easy to use end user visualization platform, but CPU pegging issues put me off, especially in browsers where it freezes the chrome.
@James,
With GWT, you can avoid runtime parsing and use GWT’s deferred binding mechanism to “compile” the processing language at compile time to JS, you could also generate ActionScript which might help with feature issues related to Canvas. If you’re interested in using a JS/Java canvas API that works fast across browsers, see http://timepedia.org/chronoscope/demo/?_force_flash , which contains a GWT Canvas implementation on-top of either the CANVAS tag, or Flash. It is exportable to JS as a minified/packed library, so doesn’t require GWT usage if you don’t want GWT.
Anonymous (May 9, 2008 at 4:03 pm)
Dear John,
Please stop releasing such great things all the time. It makes the rest of us mere mortals look like we’re lazy for just releasing one thing every couple of years.
Felipe Gomes (May 9, 2008 at 4:34 pm)
Hi John! Your work with the processing port was impressive! It is unbeliavable how good the Processing.js works, specially considering all the troubles that exist in implementing such a complex framework in a cross-platform manner. Congrats, and happy birthday too!
I had three (simple) interactive demos of Processing.org done a long time ago, and today I took the time to make them work in Processing.js, and I’ve got to say it was a pleasant work. I also coded an entirely new one only using JavaScript (no “Processing”(Java) language) to experiment with the API from the JavaScript point of view, and it indeed looks cool.
If someone would like take a look, my four demos are here: http://felipc.com/2008/05/09/processingjs/
There are two small games and two random stuff
Evan (May 9, 2008 at 4:38 pm)
Will animations with the javascript Processing API rival the Adobe Flash Platform in terms of fps, cpu demands, and filters and effects?
How do the two compare? I am experienced with Flash now but have never used Processing but have heard lots about it. I understand Processing with Java is less computer resource intensive in general than Flash.
And of course, amazing kudos to the studpendous technical achievments you have made.
Evan
Felipe Gomes (May 9, 2008 at 4:43 pm)
Hi John! Your work in the Processing port to JavaScript was impressive! It is incredible how the Processing.js works so flawlessly, considering all the troubles that might exist in implement such complex framework in a cross-platform manner.
I had three (simple) interactive demos done in Processing.org a long time ago, and today I took the opportunity to make them run in Processing.js, and I’ve got to say it was a pleasant work. I also coded a new one entirely in JavaScript (no “Processing”(Java) code) to get the feeling of the API from the JavaScript point of view, and it looks really cool.
If someone wants to see, the demos are on my page. There are two little games and two other random interactive stuff.
Liro (May 9, 2008 at 5:13 pm)
Incredible, i did not think that was humanly possible. This really pushes the boundary of js.
For one guy to code this interface alone……???
Whew…!!!!!
slig (May 9, 2008 at 5:42 pm)
thank allot! I was just thinking I needed something like this the other day as I was drawing up some program flow and venn diagrams with my pen. Great framework, and excited to start jumping in and building tools. Thanks allot for all your hard work. Interested to see where this goes.
Gustavo Cardial (May 9, 2008 at 5:47 pm)
Holy crap! You’re a truly hacker.
jive (May 9, 2008 at 5:53 pm)
Impressive stuff. This is the kind of stuff you would think only Java Applets could do. I really hope IE decides to support SVG and Canvas natively.
Dale Harvey (May 9, 2008 at 6:23 pm)
Very impressive, this looks like something that could push the adoption of canvas a bit more into the mainstream.
One small issue I have noticed (ff3) is that scrollTop is accounted for in the mouse position, so in the mouse tracking ones if you scroll, the snake or whatever will follow the amount of px you have scrolled above the actual mouse position
Ill definitely be playing around with this though, thanks
Justin Palmer (May 9, 2008 at 7:50 pm)
John,
This is amazing work and something I’ve wished I could do for a really long time! I’m glad you decided to push the limits of tomorrow’s technology instead of being defined by todays.
I’m going to look into writing a Cocoa GUI around this.
Morris (May 9, 2008 at 8:07 pm)
The source code doesn’t all display on the page if it contains a > e.g.:
http://dev.jquery.com/~john/processing.js/examples/custom/snake.html
Simplest fix is to replace the
tag with the
tag.
Looks fantastic!
Sinan Ascioglu (May 9, 2008 at 8:34 pm)
This is awesome, and this is big!!!! I can already imagine seeing it all over the web, google using for its visualizations etc..
koko (May 9, 2008 at 8:35 pm)
This is the final death blow to Java applets. Hopefully some day we can bury Flash too. Amazing.
stonemonk (May 9, 2008 at 8:38 pm)
wow… great work… perhaps consider using jsDrawing rather than canvas directly for wider browser support and better performance… not sure if this would add too much overhead or limit some features, but work looking into me thinks:
http://www.kevlindev.com/projects/jsdrawing/
management (May 9, 2008 at 9:15 pm)
Happy belated birthday, and, I would say something of value but I can’t because I’m shocked that this is so awesome. I’m telling.
Steve Parkinson (May 9, 2008 at 10:40 pm)
Impressive stuff. Now I dont have to figure out the java nightmare.
By the way, have you seen Vladimir’s Canvas 3D extension for Firefox? As well as adding support for (some of?) OpenGL ES 2.0, it also lets you run GPU shaders directly from Javascript:
<script id="shader-fs" type="x-shader/x-fragment" >
void main(void) {
gl_FragColor = vec4(0.0, 1.0, 0.0, 1.0);
}
</script>
<script id="shader-vs" type="x-shader/x-vertex">
attribute vec4 myVertex;
uniform mat4 myPMVMatrix;
void main(void) {
gl_Position = myPMVMatrix * myVertex;
}
</script>
See:
http://blog.vlad1.com/2007/11/26/canvas-3d-gl-power-web-style/
SolG (May 10, 2008 at 12:51 am)
Wow! An injection straight into my brain. Ive been looking at building an animation engine with a great language at it’s haert for a long time. Silverlight eat my shorts
John Barham (May 10, 2008 at 1:02 am)
Finally, something to justify those multi-core CPUs they’re making… ;) But seriously, awesome hack. I salute you, sir!
Kim Sullivan (May 10, 2008 at 3:45 am)
After running the Substrate demo for a bit in a tab while writing a short article, FF3b5 just crashed (withouth even trying to launch that crash reporter thingy). So, no press coverage for you, Mr. Resig!
(Just kidding ;-) I would’ve rewritten the article even if crash recovery didn’t work flawlessly. Great work!)
cluj design (May 10, 2008 at 6:38 am)
wonderfull work!
just waiting for more applications
Aaron (May 10, 2008 at 7:27 am)
daaku (May 9, 2008 at 1:36 am) :
mind boggling!
Nah, its Mind Bottling!! This is amazing :D
proppy (May 10, 2008 at 8:35 am)
Terrific!
Nice to finally know what your sneaky posts where about :)
http://ejohn.org/blog/sneaky-2/
_why the lucky stiff embedded it in a XUL app:
http://github.com/why/processor/tree/master
That can even run remotely:
http://proppy.aminche.com/why-processor/chrome/content/main.xul
proppy (May 10, 2008 at 8:38 am)
Terrific!
Nice to know that your sneaky post were about.
_why embedded it in a XUL app:
http://hackety.org/2008/05/09/someChromeForPjs.html
That can even be run remotly:
http://proppy.aminche.com/why-processor/chrome/content/main.xul
Kent Brewster (May 10, 2008 at 9:07 am)
Awesome stuff, John. (Having good results with Firefox 2.0.0.14, for what it’s worth.)
Dan (May 10, 2008 at 1:59 pm)
Rock on dude! This is something that has lots of people saying “why didnt I think of that?” … Queue the huge list of applications/extensions to come.. way to empower web devs with javascript knowledge!
Guy Fraser (May 10, 2008 at 4:13 pm)
This is better than nekked pictures of Bea Arthur. You pwn.
corey (May 10, 2008 at 6:42 pm)
*corey stands up to start the rare slow clap
clap……….. clap…………… clap
Mark (May 10, 2008 at 10:56 pm)
At least one of the demos, BezierEllipse, works on my iPhone.
Boris Reitman (May 11, 2008 at 12:16 am)
You’re a sharp guy. Checkout my project at the url.
Renars Jeromans (May 11, 2008 at 3:57 am)
This is one of the most elegant and “simple” solution to dynamic imaging on the web I have seen. I wonder what comes out of this in say 2 – 3 years.
I will definitely use your library and learn from your code.
Thanks.
Wim Leers (May 11, 2008 at 5:10 am)
Only one word:
Amazing!
Wim Leers (May 11, 2008 at 5:11 am)
Only one word:
Amazing!
Renato Formato (May 11, 2008 at 5:53 am)
Hi John,
that’s a fantastic works!!
I’ve implemented the curveVertex function along with curveTightness.
I’m not sure it works perfectly but you can give a look at the softbody demo with my modified Processing.js (http://www.fbtools.com/processing/softbody.html)
The thick is to convert a Catmull-Rom spline to a Bezier one.
Andrew (May 11, 2008 at 12:47 pm)
Awesome! Happy Birthday!
Dot. (May 11, 2008 at 1:42 pm)
The link on the frontpage goes to http://ejohn.org/blog/processingjs/ instead of http://ejohn.org/blog/processing.js/
Jeffrey (May 11, 2008 at 1:53 pm)
This has NO inherent use what so ever.
Useless javacruft. John you let the internet down.
Seriously though, keep up the good work.
charles (May 11, 2008 at 3:15 pm)
amazing! Just can’t get over how fast it is! Great job and thanks for releasing it.
jj (May 11, 2008 at 6:04 pm)
Wow! You sir, are one talented hacker. Way to score one for the cause!
Caleb (May 11, 2008 at 8:32 pm)
Fantastic stuff, John. Quick question: is there an architectural reason that there’s no loadStrings functionality? I’m trying to use it to generate on-the-fly graphs from text files in a web directory, and I keep getting a “loadStrings is not defined” error in Firebug, so I went and looked through processing.js, and I don’t see any loadStrings function. Is that intentional because it’s hard/impossible, or just a next rev feature? Either way, fantastic work, and thanks so much for sharing.
Mark M (May 12, 2008 at 5:28 am)
You have just liberated Processing.
It is great to see Processing getting so much attention, first with the Ruby wrapper [http://the-shoebox.org/apps/44] and now with this.
Dougal Campbell (May 12, 2008 at 11:16 am)
The clock demo even runs on the iPhone ;)
Andew Mallis (May 12, 2008 at 11:24 am)
Wow. Totally hardcore. You’re an animal.
nacho (May 12, 2008 at 11:50 am)
impresionante muchacho :-)
Karl (May 12, 2008 at 12:40 pm)
You r the f***ing man! I have been implementing Vector3D classes into canvas myself, but I’m not great at JS and having trouble getting things cross browser. This is exactly what I wanted, amazing! You r one sick mofo, major props!
v3ga (May 12, 2008 at 6:01 pm)
!! Bravo !!
aaron lozier (May 12, 2008 at 9:40 pm)
john – this is really incredible – it seems this could be very useful in generating creative visual reports in web apps. i am so impressed by how fast it is ! what books would you recommend to others wanting to learn the processing language? i saw this one: Processing: A Programming Handbook for Visual Designers and Artists. Do you have others you would recommend more highly?
Chris (May 13, 2008 at 12:45 am)
Patch to add support for language-specified additional shapes in beginShape, also fixes implementation of Triangle strips and endShape() call.
--- proc_orig.js 2008-05-09 19:26:39.000000000 -0500
+++ processing.js 2008-05-13 00:43:41.000000000 -0500
@@ -279,10 +279,13 @@
p.CENTER_RADIUS = 2;
p.RADIUS = 2;
p.POLYGON = 1;
+ p.QUADS = 5;
p.TRIANGLES = 6;
p.POINTS = 7;
p.LINES = 8;
p.TRIANGLE_STRIP = 9;
+ p.TRIANGLE_FAN = 4;
+ p.QUAD_STRIP = 3;
p.CORNERS = 10;
p.CLOSE = true;
p.RGB = 1;
@@ -295,6 +298,7 @@
var loopStarted = false;
var hasBackground = false;
var doLoop = true;
+ var looping = 0;
var curRectMode = p.CORNER;
var curEllipseMode = p.CENTER;
var inSetup = false;
@@ -310,7 +314,7 @@
var pathOpen = false;
var mousePressed = false;
var keyPressed = false;
- var firstX, firstY, prevX, prevY;
+ var firstX, firstY, secondX, secondY, prevX, prevY;
var curColorMode = p.RGB;
var curTint = -1;
var curTextSize = 12;
@@ -616,7 +620,7 @@
p.exit = function exit()
{
-
+ clearInterval(looping);
}
p.save = function save( file )
@@ -848,7 +852,8 @@
{
if ( curShapeCount != 0 )
{
- curContext.lineTo( firstX, firstY );
+ if ( close || doFill )
+ curContext.lineTo( firstX, firstY );
if ( doFill )
curContext.fill();
@@ -874,6 +879,8 @@
pathOpen = true;
curContext.beginPath();
curContext.moveTo( x, y );
+ firstX = x;
+ firstY = y;
}
else
{
@@ -883,13 +890,61 @@
}
else if ( arguments.length == 2 )
{
- if ( curShape == p.TRIANGLE_STRIP && curShapeCount == 2 )
- {
- curContext.moveTo( prevX, prevY );
- curContext.lineTo( firstX, firstY );
- }
+ if ( curShape != p.QUAD_STRIP || curShapeCount != 2 )
+ curContext.lineTo( x, y );
- curContext.lineTo( x, y );
+ if ( curShape == p.TRIANGLE_STRIP )
+ {
+ if ( curShapeCount == 2 )
+ {
+ // finish shape
+ p.endShape(p.CLOSE);
+ pathOpen = true;
+ curContext.beginPath();
+
+ // redraw last line to start next shape
+ curContext.moveTo( prevX, prevY );
+ curContext.lineTo( x, y );
+ curShapeCount = 1;
+ }
+ firstX = prevX;
+ firstY = prevY;
+ }
+
+ if ( curShape == p.TRIANGLE_FAN && curShapeCount == 2 )
+ {
+ // finish shape
+ p.endShape(p.CLOSE);
+ pathOpen = true;
+ curContext.beginPath();
+
+ // redraw last line to start next shape
+ curContext.moveTo( firstX, firstY );
+ curContext.lineTo( x, y );
+ curShapeCount = 1;
+ }
+
+ if ( curShape == p.QUAD_STRIP && curShapeCount == 3 )
+ {
+ // finish shape
+ curContext.lineTo( prevX, prevY );
+ p.endShape(p.CLOSE);
+ pathOpen = true;
+ curContext.beginPath();
+
+ // redraw lines to start next shape
+ curContext.moveTo( prevX, prevY );
+ curContext.lineTo( x, y );
+ curShapeCount = 1;
+ }
+
+ if ( curShape == p.QUAD_STRIP)
+ {
+ firstX = secondX;
+ firstY = secondY;
+ secondX = prevX;
+ secondY = prevY;
+ }
}
else if ( arguments.length == 4 )
{
@@ -907,23 +962,15 @@
}
}
- prevX = firstX;
- prevY = firstY;
- firstX = x;
- firstY = y;
-
-
+ prevX = x;
+ prevY = y;
curShapeCount++;
if ( curShape == p.LINES && curShapeCount == 2 ||
- (curShape == p.TRIANGLES || curShape == p.TRIANGLE_STRIP) && curShapeCount == 3 )
- {
- p.endShape();
- }
-
- if ( curShape == p.TRIANGLE_STRIP && curShapeCount == 3 )
+ (curShape == p.TRIANGLES) && curShapeCount == 3 ||
+ (curShape == p.QUADS) && curShapeCount == 4 )
{
- curShapeCount = 2;
+ p.endShape(p.CLOSE);
}
}
@@ -1044,7 +1103,7 @@
if ( loopStarted )
return;
- var looping = setInterval(function()
+ looping = setInterval(function()
{
try
{
Saj (May 13, 2008 at 4:37 am)
I agree with James..
Ramanan (May 13, 2008 at 5:31 am)
impressive. very.
ml (May 13, 2008 at 6:32 am)
great work, will use this for sure!
theStig (May 13, 2008 at 4:29 pm)
true, there are plenty of other methods to animate.. but how many of them work without downloading a plugin?
zea (May 13, 2008 at 7:17 pm)
WoW!! nice work man!
Jim Norman (May 13, 2008 at 7:17 pm)
John,
I’ve been experimenting with processing.js. Unfortunately, firebug is not available for Firefox3 beta5, and I’m having fits. I have something working, make a small change which should work, then get absolutely nothing on the screen.
How did you debug your code?
Duncan Macdonald (May 14, 2008 at 5:11 am)
YOU CAN DO MANLOVE TO ME. YOU ARE TRULY GREAT.
\o/\o/\o/
Saj (May 14, 2008 at 11:01 am)
Simply amazing..
loufoque (May 14, 2008 at 12:53 pm)
I don’t really see why you need to support the Processing language, which is actually just Java.
You can just port the API to JavaScript.
Max Kiesler (May 15, 2008 at 12:05 am)
Great job John. I’ve wanted something like this for a while now. Thanks.
Someone (May 15, 2008 at 12:52 pm)
To make the snake demo work in Opera 9.5: add width and height attributes in the img tag (isn’t that W3C standard afterall?).
Someone (May 15, 2008 at 1:00 pm)
Above fix works with the other wild demo too.
Vasudev Ram (May 15, 2008 at 1:54 pm)
Just fantastic! I had come across Processing just recently and had made a note to myself to try it out sometime. Having it on the Web makes it even better … What else can I say, except thanks for making it available. This should have tons of interesting and useful applications …
– Vasudev Ram
Schoschie (May 16, 2008 at 7:19 am)
*Gasp*
Brilliant!
*bows*
paolo (May 16, 2008 at 8:47 am)
Wow, impressive!
A small typo:
The link ‘4 custom “in the wild” demos’
points to
http://ejohn.org/apps/processing.js/examples/csutom/
while it should be
http://ejohn.org/apps/processing.js/examples/custom/
Schoschie (May 16, 2008 at 9:37 am)
Just tested all the demos in Safari 3.1.1 (4525.18) running on OS X 10.4.11
As has been stated before, all the demos work except for the ones that try to manipulate pixels directly.
Just a bit of a warning: …/topics/koch.html may freeze your browser. Safari almost froze but then displayed a “slow script” alert.
And, yes, the do hog the CPU. My 4-year old PowerBook G4 @1.25 GHz was at 100% all the time, fans on full blast :)
http://ejohn.org/apps/processing.js/examples/…
These run fine:
basic/inheritance.html
custom/molten.html
basic/creategraphics.html
basic/recursion.html
basic/distance2d.html
basic/arctangent.html
basic/random.html
basic/noisewave.html
basic/mouse2d.html
basic/bezierellipse.html
basic/storinginput.html
basic/mousefunctions.html
basic/keyboardfunctions.html
basic/clock.html
topics/handles.html
topics/wolfram.html
topics/conway.html
topics/animator.html
topics/pattern.html
topics/bouncybubbles.html
topics/collision.html
topics/reflection1.html
topics/reflection2.html
topics/spring.html
topics/springs.html
topics/reach3.html
topics/simpleparticlesystem.html
topics/flocking.html
topics/koch.html
topics/tree.html
topics/softbody.html
These DON’T run:
custom/substrate.html
custom/snake.html
basic/pointillism.html
basic/createimage.html
topics/pixelarray.html
topics/brightness.html
topics/linearimage.html
topics/scrollbar.html
topics/histogram.html
topics/mandelbrot.html
Jim Norman (May 16, 2008 at 1:23 pm)
I see none of the 3d examples work. Looking at the code, the 3d API appears not to be implemented. I’ve looked at the Canvas3D library (http://zenit.senecac.on.ca/wiki/index.php/Canvas3D_JS_Library), but am unsuccessful with that too using the latest Binary Minefield:
Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.9pre) Gecko/2008051506 Minefield/3.0pre
Have you experimented with this at all?
Jim
Ken Kennedy (May 16, 2008 at 2:36 pm)
Dude…this is crazy awesome. Thanks.
sax (May 17, 2008 at 12:25 pm)
nicely done. had never heard of ‘processing’ before but seems interesting. while experimenting with the canvas, came across a very impressive scenographic library called ‘cake’. very cool stuff and definitely worth checking out.
http://glimr.rubyforge.org/cake/canvas.html
anthony rogers (May 18, 2008 at 3:45 am)
very cool indeed- great work dude.
ehab alghazall (May 18, 2008 at 5:40 pm)
I just started trying out processing about a month ago. Now with javascript, its going to be totally awesome. Great work!
Justin Forder (May 18, 2008 at 7:14 pm)
Fantastic!
Han (May 18, 2008 at 8:49 pm)
This is just INSANELY cool! I’ve only seen the first one (the molten bar one) in Opera 9.27 and I’m already drooling.
phon (May 19, 2008 at 7:52 am)
Thank heaps John I was looking for doing processing type of graphic in flash or in adobe air. Now I can start experiment please make more tutorials.
liveoutloud2day (May 19, 2008 at 5:24 pm)
Any way this could be used for resizing an image locally before it gets uploaded (saving bandwidth & server space)? It looks awesome!
liveoutloud2day
Jonah Dempcy (May 20, 2008 at 7:00 pm)
Beautiful. I’m simply floored. Happy birthday and thanks for this great contribution!
Paulo Colacino (May 21, 2008 at 12:23 pm)
Congratulations!!! Greaaaaaaaaaaaaaaat job!!!!
Richard Quadling (May 22, 2008 at 4:21 am)
Looks great. But what’s the point?
Nano. (May 22, 2008 at 4:09 pm)
Hi John,
I found that the noTint(); function is not available. I implemented it as:
p.noTint = function noTint() {
curTint = -1;
}
I hope it is correct and useful.
Thanks,
Nano.
Beat (May 23, 2008 at 2:42 am)
Wow, really well done, John !
Opens up new perspectives in browsers !
Will Firefox 3+1 support “Processing+JQuery-Javascript” extensions natively soon ;) ?
Mona (May 24, 2008 at 3:23 pm)
that’s awesome wonderful but in some machines it tells you don’t have the latest java runtime I don’t know why and I should install the missing plugin working on FF Linux great but not as smooth as windows
Mona (May 24, 2008 at 3:29 pm)
Works at FF better than IE no way
Mona (May 24, 2008 at 3:33 pm)
Oh yeah forgot to say happy birthday
suntzu (May 24, 2008 at 9:08 pm)
With the release of http://code.google.com/p/gwt-canvas/ it would mean that this library could be good candidate for GWT. To options
a) via processing.js (via GWT JSNI)
b) via native Java (GWT)
El Artista (May 26, 2008 at 1:45 pm)
Really impresive, good work, I’ll try
paolo (May 27, 2008 at 6:25 pm)
Wow, this is awesome!!!
I would like to use this “Social Networks Library for Processing”
http://ella.slis.indiana.edu/~tohollow/SocialNetworksLibrary/
Can I use it in processing.js? Where should I place the .jar file?
Thanks!!!
David Whitten (May 29, 2008 at 12:57 am)
Hi,
Great stuff. The processing.js file did not include a few functions I needed as I was going through a few Processing books I recently bought so I added them to the js file locally. You said this might be the case. Here it is:
<code>
p.norm = function norm(value, low, high)
{
return ( (value/high) + low );
}
p.lerp = function lerp(low, high, value)
{
return ( (value * (high – low)) + low );
}
</code>
Joe (May 29, 2008 at 3:06 am)
Hey John,
Incredible work. But can someone please explain a reason for using this? Processing already runs in a browser as long as the user has Java installed which pretty much everyone has…
Except a Nintendo Wii. hmm… that gives me an idea.
But aside from that. What are the reasons for this ports existence. You can communicate to the browser and to the page through processing.
Also, Processing is not a substitute for Flash. You can do a lot of things with processing, many things much easier than in Flash. But Flash has things that make game programing much easier, things like built in dynamic arrays for example.
Brilliant work, I wish I were half as capable a programmer as you. I am still a novice programmer so I might be missing something here. Can someone please enlighten me as to why this should exist.
Very Best.
Gian Carlo Mingati (May 29, 2008 at 6:15 am)
John, you did a fantastic job!
I was trying to play with some of the samples… do the visualization of a background image only works with the three beta browser you listed? Because in FF2 no bg image is visualized/loaded? [bg = loadImage(“logo.jpg”)] even if the image has the same size of size() and it’s in the same level of the page.
GC
timothy (May 29, 2008 at 7:15 pm)
>>Processing already runs in a browser as long as the user has Java installed which pretty much everyone has..
Recent numbers I’ve seen show Java at only 56% penetration now. I no longer install it myself. Flash is about 96%.
Java has pretty much failed in the browser.
timothy (May 29, 2008 at 7:57 pm)
Just saw some other numbers. Flash at 99% and Java at 84%. Naturally, it all depends what sites are doing the looking.
I think there’s a trend of people dropping Java, though.
Adam Poit (May 29, 2008 at 9:10 pm)
Wow, this is truly impressive. I can’t wait until I see this competing with Papervision 3D.
Anna Kolesnik (May 30, 2008 at 9:11 am)
A little bit ahead of time but it’s real impressive. I hardly can wait to use it and get first results ..
Eric (May 30, 2008 at 6:44 pm)
Joe said: “But Flash has things that make game programing much easier, things like built in dynamic arrays for example.”
Java has had dynamic arrays since the first version. They are not accessed in the same way as normal arrays, but they’ve always been there. Plus, Flash’s array implementation turns out to be extremely slow – you’re even better off using a linked list than a normal array in Flash, which is a pretty serious problem, since linked lists are slow in their own way.
“What are the reasons for this ports existence. You can communicate to the browser and to the page through processing.”
Basically, people hate applets because they freeze the browser and take forever to load. Once people have Java 6u10 this should start to change and they should behave more or less just like Flash, but in the meantime, it’s great to have some way to put up simple sketches that don’t require any sort of browser plugin at all.
mattias ljungström (May 31, 2008 at 6:45 am)
I was as impressed with the Processing.js implementation as everyone else. And I naturally looked into the code to figure out how you managed to do it. As you explain, it’s all built around the browser canvas-object, and specifically the CanvasRenderingContext2D-object.
I had a look at the specification for the context object and the methods that Processing.js are using and realized that there were only about 8-10 methods needed.
So, I downloaded the JavaScript engine SpiderMonkey from Mozilla. With some inspiration from the Jiggy-project I configured and compiled it for the iphone. Then hooked it up with Processing.js, and wrote my own version of the context-object using OpenGL ES.
During this process I had to rewrite parts of Processing.js in ObjC for performance reasons.
Results? A hack of processing running on the iPhone with reasonable speed. I have uploaded a short video on youtube here: http://www.youtube.com/watch?v=0UN2iYrZmEY
Examples shown are StoringInput, BouncyBubbles (changed to use iphone accelerometer) and Substrate.
Michel Maas (June 4, 2008 at 4:21 am)
What the hell, this is some interesting stuff! When I get some time I will def. be looking into this!
Motti (June 4, 2008 at 3:12 pm)
This is very cool. On a related note, check out this online app by Philipp Lenssen (of http://blogoscoped.com/), http://bomomo.com/.
Andrew Pouliot (June 6, 2008 at 2:39 am)
Thanks so much for your contribution. I’ve been doing such things by porting to JS, but now there’s an alternative, and a mighty fine one I might add. Still can’t get over it….
Such things:
http://darknoon.com/organics/
http://darknoon.com/visuals/yellowtail/
I might just have to make a Web GUI to wrap this API….
Maybe I can convince my CS teacher to switch to processing if I show him this (you can only hope, the guy’s still using some crazy X11 custom 1995 code… eek).
Once again, thanks so much for your contribution, love to see where this goes!
Eric Pavey (June 12, 2008 at 12:22 am)
Great stuff. I’ve been a huge fan of tiddlywikis and Processing for some time. Great to see the mashup between the two ;)
Mike N. (June 13, 2008 at 12:10 pm)
Thanks John, this is really great!
There appears to be a small bug in arc(); lines are being drawn from the center to the start of the arc, contrary to the reference example:
http://processing.org/reference/arc_.html
Here’s a patch which fixes the problem:
--- processing.js.orig 2008-06-13 13:05:14.000000000 -0400
+++ processing.js 2008-06-13 13:04:47.000000000 -0400
@@ -1368,7 +1368,6 @@
curContext.beginPath();
- curContext.moveTo( x, y );
curContext.arc( x, y, curEllipseMode == p.CENTER_RADIUS ? width : width/2, start, stop, false );
if ( doFill )
Mike N. (June 13, 2008 at 12:16 pm)
Oops, I’m sorry, the previous patch was incorrect. Here’s the correct patch:
--- processing.js.orig 2008-06-13 13:05:14.000000000 -0400
+++ processing.js 2008-06-13 13:13:32.000000000 -0400
@@ -1368,7 +1368,9 @@
curContext.beginPath();
- curContext.moveTo( x, y );
+ if ( doFill )
+ curContext.moveTo( x, y );
+
curContext.arc( x, y, curEllipseMode == p.CENTER_RADIUS ? width : width/2, start, stop, false );
if ( doFill )
Carlo Giovannella (June 14, 2008 at 2:33 pm)
great project; well done !
happy birthday John !
Baudry (June 17, 2008 at 6:45 pm)
hello, I’think javascript project is interesting but how do use your script because I m’ curious i see on firefox 2.014 your html code source but i see red code on this : maybe it’ an help for you for correct bug in your script
Processing.js
Substrate
An artistic watercolor visualization by J. Tarbell.
Original Example: Substrate
// Substrate Watercolor
// j.tarbell June, 2004
// Albuquerque, New Mexico
// complexification.net
// Processing 0085 Beta syntax update
// j.tarbell April, 2005
int dimx = 250;
int dimy = 250;
int num = 0;
int maxnum = 100;
// grid of cracks
int[] cgrid;
Crack[] cracks;
// color parameters
int maxpal = 512;
int numpal = 0;
color[] goodcolor = new color[maxpal];
// sand painters
SandPainter[] sands;
// MAIN METHODS ———————————————
void setup() {
size(250,250,P3D);
background(255);
takecolor(“pollockShimmering.gif”);
cgrid = new int[dimx*dimy];
cracks = new Crack[maxnum];
begin();
}
void draw() {
// crack all cracks
for (int n=0;n1000)) {
px = int(random(dimx));
py = int(random(dimy));
if (cgrid[py*dimx+px]=0) && (cx=0) && (cy10000) || (abs(cgrid[cy*dimx+cx]-t)2) {
// crack encountered (not self), stop cracking
findStart();
//makeCrack();
}
} else {
// out of bounds, stop cracking
findStart();
//makeCrack();
}
}
void regionColor() {
// start checking one step away
float rx=x;
float ry=y;
boolean openspace=true;
// find extents of open space
while (openspace) {
// move perpendicular to crack
rx+=0.81*sin(t*PI/180);
ry-=0.81*cos(t*PI/180);
int cx = int(rx);
int cy = int(ry);
if ((cx>=0) && (cx=0) && (cy10000) {
// space is open
} else {
openspace=false;
}
} else {
openspace=false;
}
}
// draw sand painter
sp.render(rx,ry,x,y);
}
}
class SandPainter {
color c;
float g;
SandPainter() {
c = somecolor();
g = random(0.01,0.1);
}
void render(float x, float y, float ox, float oy) {
// modulate gain
g+=random(-0.050,0.050);
float maxg = 1.0;
if (gmaxg) g=maxg;
// calculate grains by distance
//int grains = int(sqrt((ox-x)*(ox-x)+(oy-y)*(oy-y)));
int grains = 64;
// lay down grains of sand (transparent pixels)
float w = g/(grains-1);
for (int i=0;i
// Substrate Watercolor
// j.tarbell June, 2004
// Albuquerque, New Mexico
// complexification.net
// Processing 0085 Beta syntax update
// j.tarbell April, 2005
int dimx = 250;
int dimy = 250;
int num = 0;
int maxnum = 100;
// grid of cracks
int[] cgrid;
Crack[] cracks;
// color parameters
int maxpal = 512;
int numpal = 0;
color[] goodcolor = new color[maxpal];
// sand painters
SandPainter[] sands;
// MAIN METHODS ———————————————
void setup() {
size(250,250,P3D);
background(255);
takecolor(“pollockShimmering.gif”);
cgrid = new int[dimx*dimy];
cracks = new Crack[maxnum];
begin();
}
void draw() {
// crack all cracks
for (int n=0;n1000)) {
px = int(random(dimx));
py = int(random(dimy));
if (cgrid[py*dimx+px]=0) && (cx=0) && (cy10000) || (abs(cgrid[cy*dimx+cx]-t)2) {
// crack encountered (not self), stop cracking
findStart();
//makeCrack();
}
} else {
// out of bounds, stop cracking
findStart();
//makeCrack();
}
}
void regionColor() {
// start checking one step away
float rx=x;
float ry=y;
boolean openspace=true;
// find extents of open space
while (openspace) {
// move perpendicular to crack
rx+=0.81*sin(t*PI/180);
ry-=0.81*cos(t*PI/180);
int cx = int(rx);
int cy = int(ry);
if ((cx>=0) && (cx=0) && (cy10000) {
// space is open
} else {
openspace=false;
}
} else {
openspace=false;
}
}
// draw sand painter
sp.render(rx,ry,x,y);
}
}
class SandPainter {
color c;
float g;
SandPainter() {
c = somecolor();
g = random(0.01,0.1);
}
void render(float x, float y, float ox, float oy) {
// modulate gain
g+=random(-0.050,0.050);
float maxg = 1.0;
if (gmaxg) g=maxg;
// calculate grains by distance
//int grains = int(sqrt((ox-x)*(ox-x)+(oy-y)*(oy-y)));
int grains = 64;
// lay down grains of sand (transparent pixels)
float w = g/(grains-1);
for (int i=0;i
Dan Imal (June 18, 2008 at 10:57 pm)
My god. This is absolutely incredible. Beautiful. I don’t know how to express it. Its level of thoroughness is absurd. It’s humiliating too. I’m just getting into canvas and thought, “it would be nice if I adapted the processing api to canvas” and then I find this! Well, at least I don’t have to actually do it. But then again, I would never have done it nearly as well.
Eli Cochran (June 22, 2008 at 4:49 pm)
John,
Have you seen this page that does visual representations of DOM using the Processing language?
http://www.aharef.info/static/htmlgraph/?url=http%3A%2F%2Fejohn.org%2F
Do you think that you could pull that off using Processing.js?