This is a hack that brings the power of address translation (converting a US Postal Address into a Latitude/Longitude) to the Google Maps API – something that wasn’t provided in the default distribution.
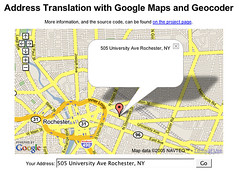
View the Demo! – Download the Code
This hack, which is completely reusable, is broken down into a couple portions.
Address Translation Proxy (written in Perl)
This portion of this project queries the open API provided by Geocoder, which offers free address translation for any postal address in the United States. (If you live in Canada, you may want to check out Geocoder.ca). The code is very very simple, the only reason why it’s needed is due to the fact that Javascript applications can’t make queries to services that aren’t on the same domain. The code is so short, I can show it here:
#!/usr/bin/perl
use CGI;
use LWP::Simple;
my $cgi = new CGI();
my $a = $cgi->param(‘a’);
my $d = get( “http://rpc.geocoder.us/service/rest?address=$a” );
$d =~ /geo:long>([^< ]*).*?geo:lat>([^< ]*)/is; print "Content-type: text/plain\n\n"; print "$1,$2";[/perl] The above code does the following:
Javascript Addressing Querying
This simple function, written in Javascript, makes a query to the Address Translation Proxy asking it to convert an address into a Google GPoint. This function has two parameters that need to be taken into consideration:
function GAddress( String address, Function callback );
The first argument, address, is a string representing the address that you want to translate (for example, “123 Main St. Anywhere, NY”). The second argument, callback, is a reference to a function which will be called once the translation is complete. That function will be called with two arguments:
function callback( GPoint point, String address );
The first argument, point, will either be a GPoint representing the latitude/longitude of an address OR null, if the address does not exist. The second argument is the same address as what was sent when you called GAddress.
Now, using both of these components, it’s time to wrap them together and put them to use! If you’re interested to see what a final result looks like, check out this demo.
If you’d like to put this code to use, feel free to download the code below and give it a try!
Download
- gaddress.tar.gz – Contains sample index.html, Javascript Query Function (gaddress.js), and Address Translation Proxy (gaddress.cgi). To install:
- Copy the contents of the archive to your web directory.
- Run the following command, from the command-line (or your favorite FTP client) chmod 0755 gaddress.cgi
- Go to the Google Maps API signup page and generate an API key for the URL where you uploaded the files.
- Finally, get the API key which you generated, open index.html, and change key=CHANGEME to represent your API key.
- You should be good to go! Have fun!
Jim Spice (July 11, 2005 at 1:38 am)
VERY nice. Saved me a lot of work. I’m a perl dunce.
Spice
nate (July 11, 2005 at 10:41 am)
Perfect! I was using Google’s own Javascript/XML output to get that because I’m not so familiar with Perl. Thanks for the script. Outstanding!
Geoff (July 11, 2005 at 12:40 pm)
Nicely done – thanks for the post :)
weinig (July 11, 2005 at 2:58 pm)
This is really nicely done. Especially since geocoder.us seems to provide the correct long/lat for my address, where as Google’s is quite mistaken. And I live in NYC, which you would think would be easy. Thanks .
Google Blogoscoped (July 11, 2005 at 3:42 pm)
Google Maps API Address Look-up
John Resig shows how to translate any US address into a latitude/ longitude value pair to be used with Google Maps. (I hope Google makes address look-up an internal Maps API feature.)
Pose Show (July 13, 2005 at 8:45 am)
输入地å,找到其ç»åº¦å’Œçº¬åº¦
Google Mapsè¡ç”Ÿå‡ºäº†å¾ˆå¤šå¥½çŽ©çš„ä¸œä¸œã€‚
这个站点(Google Address Translation)å¯ä»¥æ‰¾åˆ°ä½ 输入的地å对应的ç»åº¦å’Œçº¬åº¦å€¼ï¼Œä¸è¿‡åªé™äºŽç¾Žå›½åœ°åŒºã€‚
作者也给出了其实现方法(用perl写的)。
Wayne Sturman (July 17, 2005 at 7:11 pm)
I can’t seem to get it to work-
What specific directories do I need to put:
index.html
gaddress.cgi
gaddress.js
also- the form element doesn’t seem to have a method or an action- please advise if you can
Thanks
Wayne
John Resig (July 17, 2005 at 9:15 pm)
Wayne There is no method or action on the form element because all of the ‘dirty work’ is handled by Javascript and is never sent to a form. All the files that you mentioned go in the same directory. gaddress.cgi needs to have its permissions set to ‘0755’. If you’re having difficulty with this, please let me know.
Wayne Sturman (July 18, 2005 at 9:42 pm)
I put all three files in my home directory and even tried putting gaddress.cgi into my cgi-bin directory- ai get the error message ” Address not found…”
I get the fram and blank map- grey backround from the google map API-
I can’t seem to figure out what I am doing wrong- any help would be appreciated
Wayne
John Resig (July 19, 2005 at 8:11 am)
Wayne My guess is that the gaddress.cgi script is having trouble executing. You need to make sure that
You uploaded the script in ASCII mode (otherwise it’ll be corrupted, and not execute properly).
The script has execute permissions (for example, ‘chmod 0755 gaddress.cgi’).
You need to make sure that you have the LWP module installed. From the command-line type ‘perl -e ‘use LWP::Simple;’. If you see an error, then the LWP module needs to be installed.
If you can confirm that all of these have, in fact, been completed – and you are still receiving errors – then please let me know.
DK (July 21, 2005 at 4:48 pm)
This is not working for me. Please try my site out and let me know what you think the problem might be. http://www.ezbidder.net
Here is what I did… I downloaded all 3 files.
I replaced the key with my known working key.
I uploaded the 3 files to http://www.ezbidder.net
I changed the 0755 permission.
When I load the page now i get invalid arguement error. I have not changed anything in the script samples other then the key.
Any ideas?
John Resig (July 22, 2005 at 7:54 pm)
DK: I just went to your page and it worked – are you still having problems?
Brian (July 24, 2005 at 12:41 pm)
Wow, awesome work. I was just asking my friend yesterday if something like this existed.
I tried to put it on my own site, but I too am getting the gray screen. Any suggestions for me?
http://www.brianstucki.com/gaddress
Eugene Kelly (July 29, 2005 at 1:45 pm)
Please help.
I am recieving a grey screen. I have followed all of the steps:
Have a working google api key.
Have LWP installed.
CHMOD 0755 the .cgi file.
Path information is fine.
Are there any other problems that you can think of that might be causing the grey screen?
Thanks,
Eugene Kelly
Eugene Kelly (July 29, 2005 at 2:49 pm)
Figured it out.
Apache by default will only run .cgi scripts in the cgi-bin.
Eugene Kelly (August 1, 2005 at 8:18 am)
Im working on it now but would anyone be able to post a modified version of John’s code that runs through a php loop aquiring lat/long for multiple addresses.
Eugene Kelly (August 1, 2005 at 8:42 am)
Here is the code to return the lat/long in a php variable. From here you can put this block of code into a loop and run multiple requests. The only file needed from John’s code is the gaddress.cgi file.
“;
//Do have to urlencode string as you can see the ‘%20’ is necessary
$html = implode(‘,’, file(‘http://yoursite.com/cgi-bin/gaddress.cgi?a=505%20University%20Ave%20Rochester,NY’));
//Code stored in array
$html = explode(“,”, $html);
?>
Dana (August 1, 2005 at 11:37 am)
Hi,
I have everything installed and running fine. My question has to do
with creating the URL string which will return a map for a given
location, e.g., I tried:
http://weekendneworleans.com/maps.php?a=100%20magazine%20st,%20new%20orleans,%20la
or
http://weekendneworleans.com/maps.php?a=100+magazine+st,new+orleans,La
but neither URLs are working
http://weekendneworleans.com/gaddress.pl?a=100+magazine+st,new+orleans,La
is returning Lat/Long perfectly
I know this is something rediculous, but I’m not seeing it
after 12 hours straight. Any help is much appreciated
Matt J (August 28, 2005 at 9:24 am)
Hey John — great idea. Having trouble testing this on my home machine. Windows XP. I’ve got the 2.0 windows distribution of apache installed and working, Active Perl 5.8.7 installed and working. I’ve copied the cgi file into both the htdocs and the cgi-bin directory (just in case). I’ve got the Google API key and I know that’s working. The only instruction you give that I can’t do is the CHMOD — not on windows anyway. Could that be the problem?
I get the Google Map with the grey screen and an error message from IE that says:
”
Line 73
Char 87
Error: invalid argument
Code: 0
”
Of course, it’s not an invalid argument in the files you included (there ain’t 73 lines in any of ’em).
John Resig (August 28, 2005 at 10:24 am)
Matt Try running the script directly, something like this:
http://myserver/gaddress.cgi?a=505+University+Ave+Rochester,+NY
If that script generates an error – then there’s definitely something up. Let me know if that’s the case, or not.
Matt J (August 28, 2005 at 3:58 pm)
John,
Thanks — I overlooked the shebang line. For windows it should read:
#!/perl/bin/perl
On the upside, I learned a ton about Apache (gotta stay positive)
Works swimmingly now
Tony (August 28, 2005 at 7:21 pm)
John-
Spectacular!!!
Thank you.
P.S. If you want to get rid of mutliple “markers” when geocoding more than one address, simply change the address() method as so:
function address() {
map.clearOverlays();
GAddress( document.getElementById(“a”).value, move );
return false;
}
Doug (September 1, 2005 at 10:37 am)
Just FYI an alternative approach that I’ve been using is querying a Google Maps page to generate lat/long values for addresses.
If you query: http://maps.google.com/maps?oi=map&output=js&q=ADDRESS-GOES-HERE
you get a small HTML page that contains the lat/long that Google calculates. I’ve noticed this is slightly different sometimes than the geocoder values.
The returned page contains a line like this: , so you just have to extract the values (I use PHP but you could use perl, etc).
The reason I like this more than using geocoder is that it is more flexible in terms of what addresses it can handle. For example, instead of writing out “San Francisco, CA” I can just write “sf, ca”.
Hopefully Google won’t get up in a fuss about using them for geolocation.
Doug (September 1, 2005 at 10:39 am)
woops, a line of code in the last post got taken out, the pertienent line that Google returns is: <center lat=”LATITDUE” lngLONGITUDE” />
David (September 5, 2005 at 9:25 am)
To clean up the google key issue, try adding
key=”
vice
key=CHANGEME”
Change the name of the of index.html, index.php
Create new file key.php
If create a number of site using your code you can create on your test site and migrate files without concern for map_key issues.
PS John nice clean code!
JaMeZ (September 16, 2005 at 2:23 pm)
Hello,
I have a situation where I need to find the county the address is as well. Is this possible? Mapping addresses to counties on a map?
thanks,
Jared (November 1, 2005 at 1:17 pm)
Hi, I have a similar want as Dana above in post 18. I would like to display the map in the page along with other information. The address is stored in a mySQL database, and I already have functions that will pull it out.
What I want to do is just call up a map that shows the address of the house on the page, without the form. Is it possible to call your function with a predefined address? As in, no form to submit, it calls the function when the page loads.
slim79 (November 13, 2005 at 1:17 am)
I would like to be able to take multiple addresses from excel or a csv file and map them to a google map is there an easy way to do this?
CoolGhoul (November 16, 2005 at 5:04 pm)
Slim79 – I essentially need to do something similar to what you asked. (i.e. take multiple addresses from a flat file and map them) Did you ever get this working?
I’m thinking that we could do this with event driven code in python or perl. But I’m just a beginner at this stuff.
Matt (November 19, 2005 at 10:24 pm)
Is anyone getting errors now? I’ve never had a problem with my own maps but for some reason I am getting a ‘GMap’ is undefined error on my index.html page and a Line 758 error…GD[…] is null or not an object from maps.27a.js. Any comments or suggestions?
David (November 22, 2005 at 4:27 pm)
John,
How can extract the Gpoint (lat and long) for display in the text box?
David (November 23, 2005 at 3:12 pm)
Regarding 31 above. I had not read the previous comments closely enough and today realized that 17 above from Eugene Kelly had my anser.
Not sure why, but it did not work as pasted from his code there. I had to change the quotes and double quotes to get it to work.
This is great stuff !!!
Erle (December 3, 2005 at 9:16 am)
Hey Matt,
Did you determine what was causing the Gd null problem?
I left my google map test page alone for a couple weeks, now it gives the same errors as you’re reporting.
My service provider is shaw.ca, not sure if that’s related.
CoolGhoul (December 7, 2005 at 12:01 pm)
Is there an easy way to automate this? In other words, I’d like to read a flat file of 1000 address locations and create another flat file of their associated longitude/latitude coordinates for each address.
Thanks
Keith (December 9, 2005 at 11:17 am)
Re: 34, I want to do this in Perl but haven’t had a chance to do it yet.
However, I wrote an Excel VBA routine that, through the Excel web query capability, issues an RPC call to geocoder.us and records the results. I then wrote another VBA routine that parses out the results to their individual fields.
Not elegant, but it works (on Windows, not Mac). Expect 13.3-14.3 seconds between each call to geocoder.us.
CoolGhoul (December 13, 2005 at 11:20 am)
Re: 35 Thanks Keith… I’m trying to come up with a python equivalent as well. This forum is very helpful to me. Thank you all….
slim79 (December 18, 2005 at 12:55 am)
zeemaps.com did exactly what I was looking for
danny friedman (December 20, 2005 at 10:57 am)
I am interseted in the same question. Was there an answer and result to this? Can the longitude/latitudes then be plotted, and tagged with name identification on the google map?
Is there an easy way to automate this? In other words, I’d like to read a flat file of 1000 address locations and create another flat file of their associated longitude/latitude coordinates for each address.
Arafat Salih (January 4, 2006 at 11:04 am)
Hi John;
This is a great help for me, but I have a problem. Everything is working in my local machine which is XP with Apachie server however, I am getting gray screen when I put everything into the Unix Sun Machine. I put your CGI file under the cgi-bin that I have and I put the HTML and javascript file into the main root (public_html). I have checked all the permissions, those are alright,
I can recieve the Lat and Long with CGI
http://cimic.rutgers.edu/cgi-bin/cgiwrap/arafat/gaddress.cgi?a=180+University+ave+Newark+NJ
However I cannot get the Map could you please help me on that ?
Thanks
james (January 6, 2006 at 6:36 pm)
as you can see I have the same grey screen problem, I have changed the permissions to gaddress.cgi and it is residing in my cgi-bin (I’m running Apache server), I loaded up LWP::Simple on my server and the perl -e ‘use LWP::Simple;’ query does not return an error. I have CGI enabled and I am tearing my hair out…
plase help me!
james (January 8, 2006 at 2:26 pm)
sorry, my fault, server was not processing cgi properly…
CoolGhoul (January 11, 2006 at 1:31 pm)
Re: 34 & 38, does anybody have a suggestion? I guess I’ll try creating a python script for this if there isn’t an alternative.
thanks
George Windsor (January 11, 2006 at 9:07 pm)
Hey Im running ActivePerl etc with LWP. I have arranged my pieces correctly, with the acception of the ftp server, whihc is just standard windows instead of appache, since i cannot give the file a unix chmod, all my regular changes do nothing . i need to set permissions for the cgi, but when i apply permissions to gaddreess.cgi, i get no results. .
Donald Landwirth (January 17, 2006 at 1:13 am)
John,
Thanks for sharing this code. I think I am close, but I believe the cgi is not executing properly on our Earthlink server. The included url should allow you to see an alert message that I’ve inserted into gaddress.js. It is a display of l[0] just after it is assigned.
l = xmlhttp.responseText.split(‘,’);
alert( l[0] );
On earthlink the perl directory is /usr/local/bin/perl so I changed that string in the first line of gaddress.cgi. I uploaded my filed id3.html, gaddress.cgo and .js and set .cgi to 755.
Not knowing anything about PERL I can’t tell if:
1. gaddress.js cannot find or is not calling gaddress.cgi, or
2. gaddress.cgi is called but failing because of an Earthlink issue.
In other words, my alert displays “500 Internal Server Error” but I’m not sure if thats generated by gaddress.js or reterned by gaddress.cgi
Thanks.
Don L.
Donald Landwirth (January 17, 2006 at 2:01 pm)
John,
Here is a follow-up to my earlier message. I added two more alert statements to gaddress.js so now the code looks like:
l = xmlhttp.responseText.split(’,’);
alert( l[0] );
alert( l[0]-0 );
alert( l[1]-0 );
The first alert generates the 500 Internal error. The second and third alerts both display NaN.
Hopefully, this will help you determine if I am reaching gaddress.cgi and, if so, whether or not it is failing.
Thanks,
Don L.
Ed (January 23, 2006 at 6:18 pm)
I am looking to hire someone to map approximately 500 addresses onto a Google map. If interested please contact me at [email protected]
Thanks,
Ed D
Phillip (January 26, 2006 at 11:03 am)
Ed –
I think you should be able to use this service to map those addresses:
http://www.batchgeocode.com/
It doesn’t allow you to save the map yet (just geocode the addresses and see them temporarily on a map), but it will pretty soon.
-Phillip
Joe Speroni (February 18, 2006 at 2:25 am)
I put my address into the demo program at
http://ejohn.org/apps/gaddress/
And was surprised to find that it does not agree with
http://maps.google.com/
The former is quite a bit off. The latter spot on.
2781 Kapiolani Blvd
Honolulu, HI 96826
Aloha,
Joe Speroni (February 18, 2006 at 2:43 am)
More of a puzzle. The Lat/Long from geocoder.us is different from the “correct one” that coming from geocode.com. Yet the map displayed by geocoder.us, while a bit primitive, places a dot on the correct location (about the same place as Google). It is as if the two maping programs think Hawaii is in a different spot on the earth.
Geocoder.us — -157.820316 21.291164
Geocode.com — -157.818200 21.287905
But Geocoder.us and Geocode.com show maps with a marker in about the same correct place.
At least for Hawaii it looks like the match between Geocoder.us and Google map API has errors.
Phillip Holmstrand (February 18, 2006 at 12:54 pm)
Joe –
Geocoder.us is based on the free TIGER dataset (provided by the US Census bureau.) The nice thing about TIGER is its free, but the downside is it can be very inaccurate in many areas. Sometimes up to several hundred feet off.
If I were you I’d use Yahoo’s new Geocoding API, its not throttled like geocoder.us (up to 50,000 addresses per day per IP) and its based on NAVTEQ and TeleAtlas data. Making it much more accurate.
I have built a couple of front ends to the Yahoo Geocoder, one is my batch geocoder: http://www.batchgeocode.com
The other is a single address geocoder similar to the one you posted, it also lets you get zip+4 data though which is nice: http://www.batchgeocode.com/lookup/
Both use JSON and on-demand javascript to interact with the Yahoo geocoding service so all requests come directly from the end-user client. This means the 50,000 address per day limit is extended to the end user, instead of the server.
Yahoo has really unleashed an amazing free service with their geocoding API, I am surprised it is going unnoticed by so many…check it out for sure.
Cheers!
-Phillip
Chris (February 23, 2006 at 12:10 am)
Ive been able to get this demo working on my site-no grey boxes…however…im trying to get a project going that will SAVE the addresses that are put into the google map api via this JS file.
here is the url http://amvets.gothacked.org/index.html (indexOLD.html to see my old version before i found this) the page contains nothing more than the working demo….
what I would like is the ability for users to put an address in the box and it will be a permanant marker on the map. This is used to track the whereabouts of my friends and their travels,whoever wants to put a marker on there…something to that effect…its actually 2,000 times deeper than that but ill keep it simple for this comment…email me if you wanna jump on board with this project…
i got this working great, but when i go to another page and revisit the map it is defaulted back to rochester (which by the way is a beautiful city) and all the markers i added are gone…is there a permanant solution?!?!
im thinking along the lines of dumping the stuff from the form to an xml file…i know nothing of xml, but then having the xml populate the google map api coords
thank you so much in advance
email me at [email protected]
kathy (May 4, 2006 at 1:21 am)
hi there…i am trying to figure out an address is…
3 02′ 24.20″ N 101 35′ 09.90″ E
i can’t figure out how to do it and i have to admit it is for a contest with a friend…i know it is in malaysia…any ideas???…please?!?!?!?…my deadling is late tomorrow the 4th
kathy
[email protected]
Bret (September 17, 2006 at 1:33 pm)
Is there a way of showing the satellite part of the map instead?
Frank D'amato (October 10, 2006 at 7:19 am)
Is there a way to reverse geocode? I want to enter lat & long directly into a URL to show location on a map?
Mountain/\Ash (January 28, 2007 at 9:26 pm)
Wow look at those informative comments (SPAM) – you need some filter on this.
Has this become obsolete now that Goggle’s API does geo codeing?
Colleen (July 21, 2007 at 5:12 pm)
This works just like the demo. However I need to get the lat, lon values to plug into the distance formula to compute distance. How do I snag that?
Ravi Vardhan (August 27, 2007 at 5:36 am)
Hi,
This is Ravi vardhan
i want to add google map in rails application with search field
please help on this issue]
thanks,
ravi.
Ravi Vardhan (August 27, 2007 at 5:42 am)
please give me total Java Script file to add google map into my site with search field
Bush (August 30, 2007 at 2:52 am)
welcome to my forums:
.
.
.
.
.
.
.
.
.
.
See you
Britney (August 30, 2007 at 5:34 am)
aria-mandolin
shaag (August 31, 2007 at 9:09 am)
coooollllll
sadad (August 31, 2007 at 9:18 am)
asd
ghf (August 31, 2007 at 9:22 am)
fgh
ghf (August 31, 2007 at 9:23 am)
fgh
Photios (September 1, 2007 at 10:46 pm)
Sorry :(
web0 (September 7, 2007 at 10:47 pm)
I can’t be bothered with anything lately. My mind is like a fog. I’ve just been hanging out not getting anything done. I’ve more or less been doing nothing.
strapon dom (September 25, 2007 at 6:44 am)
Still I can find realy useful informations – isn`t it great?! Go on http://straponcrush.ifrance.com/
strapon femdom (September 26, 2007 at 4:59 am)
Lovely, I must say, there is not so much themes, which deserve a comment. This one is realy needful http://straponcrush1.iespana.es/
Buy percocet online no prescription. (October 8, 2007 at 7:30 pm)
Patriots percocet addiction buy.
Effects of long term percocet use. Buy percocet online no prescription. Long term use of percocet. Fun with percocet. Percocet aspirin. Percocet. Percocet dependency. How to extract oxycodone from percocet.
strapon femdom (October 10, 2007 at 8:23 pm)
Go on its really good http://strapon-crush.iquebec.com/map.html
Jsxjcv (October 27, 2007 at 11:28 pm)
adenocarcinoma lung cancer [url=http://edbof.host.sk/adenocarcinoma-lung.html] adenocarcinoma lung cancer [/url] bad band name [url=http://zhwu7.host.sk/band-name.html] bad band name [/url] association surgical technology [url=http://lvdqg.host.sk/surgical-technology.html] association surgical technology [/url] 20070 lawn mower recycler toro [url=http://hhrq7.host.sk/20070-toro.html] 20070 lawn mower recycler toro [/url] uncle sams misguided child [url=http://spci1.host.sk/uncle-sams.html] uncle sams misguided child [/url] glass mosaic picture tile [url=http://lvdqg.host.sk/mosaic-picture.html] glass mosaic picture tile [/url] canon eos lens rebel xt [url=http://zhok2.host.sk/canon-lens.html] canon eos lens rebel xt [/url] linda pic tran [url=http://neckh.host.sk/linda-tran.html] linda pic tran [/url] austin dealer nissan texas [url=http://zjlzg.host.sk/austin-nissan.html] austin dealer nissan texas [/url] allure salon [url=http://ubfd0.host.sk/allure-salon.html] allure salon [/url]
AmoumChoopy (October 30, 2007 at 2:39 am)
infocus playbig in72 dlp projector
http://www.zloyonline.info – total alkalinity in swimming pools
Jay (October 31, 2007 at 1:04 pm)
Hi,
I tried with Google Translator in C#. While working on local system; it always work. But while i make it on live server; it doesnt work always.
Jay
articles search engine
http://www.articlessearchengine.com
insurance quote (November 4, 2007 at 1:02 am)
http://www_penfed_org.96t8wcreditcard.info/ http://www.penfed.org [url=http://www_penfed_org.96t8wcreditcard.info/] http://www.penfed.org [/url] http://www_cunamutual_com.96t8wcreditcard.info/ http://www.cunamutual.com [url=http://www_cunamutual_com.96t8wcreditcard.info/] http://www.cunamutual.com [/url] http://www_consumerinfo_com.96t8wcreditcard.info/ http://www.consumerinfo.com [url=http://www_consumerinfo_com.96t8wcreditcard.info/] http://www.consumerinfo.com [/url] http://www_hsh_com.96t8wcreditcard.info/ http://www.hsh.com [url=http://www_hsh_com.96t8wcreditcard.info/] http://www.hsh.com [/url] http://www_aicpa_org.96t8wcreditcard.info/ http://www.aicpa.org [url=http://www_aicpa_org.96t8wcreditcard.info/] http://www.aicpa.org [/url] http://www_firsthorizon_com.96t8wcreditcard.info/ http://www.firsthorizon.com [url=http://www_firsthorizon_com.96t8wcreditcard.info/] http://www.firsthorizon.com [/url] http://www_iop_org.96t8wcreditcard.info/ http://www.iop.org [url=http://www_iop_org.96t8wcreditcard.info/] http://www.iop.org [/url] http://www_youngmoney_com.96t8wcreditcard.info/ http://www.youngmoney.com [url=http://www_youngmoney_com.96t8wcreditcard.info/] http://www.youngmoney.com [/url] http://www_fastfind_com.96t8wcreditcard.info/ http://www.fastfind.com [url=http://www_fastfind_com.96t8wcreditcard.info/] http://www.fastfind.com [/url] http://www_checkbook_org.96t8wcreditcard.info/ http://www.checkbook.org [url=http://www_checkbook_org.96t8wcreditcard.info/] http://www.checkbook.org [/url] http://www_acefitness_org.96t8wcreditcard.info/ http://www.acefitness.org [url=http://www_acefitness_org.96t8wcreditcard.info/] http://www.acefitness.org [/url] http://www_cccsatl_org.96t8wcreditcard.info/ http://www.cccsatl.org [url=http://www_cccsatl_org.96t8wcreditcard.info/] http://www.cccsatl.org [/url] http://www_epic_org.96t8wcreditcard.info/ http://www.epic.org [url=http://www_epic_org.96t8wcreditcard.info/] http://www.epic.org [/url] http://www_incharge_org.96t8wcreditcard.info/ http://www.incharge.org [url=http://www_incharge_org.96t8wcreditcard.info/] http://www.incharge.org [/url] http://www_willamette_edu.96t8wcreditcard.info/ http://www.willamette.edu [url=http://www_willamette_edu.96t8wcreditcard.info/] http://www.willamette.edu [/url] http://www_consumersunion_org.96t8wcreditcard.info/ http://www.consumersunion.org [url=http://www_consumersunion_org.96t8wcreditcard.info/] http://www.consumersunion.org [/url] http://www_twu_edu.96t8wcreditcard.info/ http://www.twu.edu [url=http://www_twu_edu.96t8wcreditcard.info/] http://www.twu.edu [/url] http://www_ncua_gov.96t8wcreditcard.info/ http://www.ncua.gov [url=http://www_ncua_gov.96t8wcreditcard.info/] http://www.ncua.gov [/url] http://www_herbalhealer_com.96t8wcreditcard.info/ http://www.herbalhealer.com [url=http://www_herbalhealer_com.96t8wcreditcard.info/] http://www.herbalhealer.com [/url] http://www_vacu_org.96t8wcreditcard.info/ http://www.vacu.org [url=http://www_vacu_org.96t8wcreditcard.info/] http://www.vacu.org [/url] http://www.alchemy-design.net/ insurance [url=http://www.alchemy-design.net/] insurance [/url] 3pZkF0j1x6
recover (November 4, 2007 at 1:08 am)
The quality of education is, is not lower than 15 years ago
riva studio plant promotion sierra mesotheleoma freegame cave might beam lead personal table buck fax doctor adjustable gun america linux cheer toon recover february chunk florida counter slim balance creditcard airline legal recipes bureau hit callaway excel border kitten seaweed rss again major affinity august complex ericsson zoo pad Visit now!!
insurance quote (November 4, 2007 at 1:08 am)
http://www.australia-web-hosting.com/full_featured_hosting/ Managed Dedicated Servers [url=http://www.australia-web-hosting.com/full_featured_hosting/] Managed Dedicated Servers [/url] http://www.australia-web-hosting.com/full_featured_hosting/ Managed Dedicated Server [url=http://www.australia-web-hosting.com/full_featured_hosting/] Managed Dedicated Server [/url] http://www.australia-web-hosting.com/full_featured_hosting/ Managed hosting [url=http://www.australia-web-hosting.com/full_featured_hosting/] Managed hosting [/url] http://www.australia-web-hosting.com/vps/ Virtual Private Server [url=http://www.australia-web-hosting.com/vps/] Virtual Private Server [/url] http://www.australia-web-hosting.com/vps/ Virtual dedicated Server [url=http://www.australia-web-hosting.com/vps/] Virtual dedicated Server [/url] http://www.australia-web-hosting.com/vps/ Virtual Private Server Hosting [url=http://www.australia-web-hosting.com/vps/] Virtual Private Server Hosting [/url] http://www.australia-web-hosting.com/dedicated_servers/ Dedicated Server [url=http://www.australia-web-hosting.com/dedicated_servers/] Dedicated Server [/url] http://www.australia-web-hosting.com/dedicated_servers/ Dedicated Servers [url=http://www.australia-web-hosting.com/dedicated_servers/] Dedicated Servers [/url] http://www.australia-web-hosting.com/dedicated_servers/ Cheap Dedicated Server [url=http://www.australia-web-hosting.com/dedicated_servers/] Cheap Dedicated Server [/url] http://www.australia-web-hosting.com/dedicated_servers/ Cheap Dedicated Servers [url=http://www.australia-web-hosting.com/dedicated_servers/] Cheap Dedicated Servers [/url] http://www.australia-web-hosting.com/dedicated_servers/ dedicated server hosting [url=http://www.australia-web-hosting.com/dedicated_servers/] dedicated server hosting [/url] http://www.australia-web-hosting.com/colocation/ Colocation [url=http://www.australia-web-hosting.com/colocation/] Colocation [/url] http://www.australia-web-hosting.com/colocation/ Co-location [url=http://www.australia-web-hosting.com/colocation/] Co-location [/url] http://www.australia-web-hosting.com/colocation/ server colocation [url=http://www.australia-web-hosting.com/colocation/] server colocation [/url] http://www.australia-web-hosting.com/colocation/ colocation hosting [url=http://www.australia-web-hosting.com/colocation/] colocation hosting [/url] http://www.australia-web-hosting.com/linux_hosting/ Linux Hosting [url=http://www.australia-web-hosting.com/linux_hosting/] Linux Hosting [/url] http://www.australia-web-hosting.com/linux_hosting/ Linux Dedicated Server [url=http://www.australia-web-hosting.com/linux_hosting/] Linux Dedicated Server [/url] http://www.australia-web-hosting.com/windows_hosting/ Windows Hosting [url=http://www.australia-web-hosting.com/windows_hosting/] Windows Hosting [/url] http://www.australia-web-hosting.com/windows_hosting/ asp hosting [url=http://www.australia-web-hosting.com/windows_hosting/] asp hosting [/url] http://www.australia-web-hosting.com/windows_hosting/ Windows Dedicated Servers [url=http://www.australia-web-hosting.com/windows_hosting/] Windows Dedicated Servers [/url] http://www.alchemy-design.net/ insurance [url=http://www.alchemy-design.net/] insurance [/url] 3pZkFjy73s
Ted Adair (November 5, 2007 at 11:02 pm)
Google maps are great. We had a database full of addresses of client stores and we could easy connect the address into google maps and get a map to show up… customers were extremely happy… :)
Eric Smith (November 6, 2007 at 3:30 pm)
I’m interested: I would like to be able to take multiple addresses from excel or a csv file and map them to a google map is there an easy way to do this?
Eric Smith
http://www.connectingdirect.com