This is the final part of a week long series featuring code for the Google Maps API.
For the last day of this series (don’t worry, this isn’t the end of me having fun with Google Maps – there’s still much more to come!) I decided to implement two things.
The first new feature is a sane version of the afformentioned Mouse Wheel Zooming. This version is completely streamlined, tied straight into the GEvent API, and isn’t limited to only zooming.
Moving the mouse wheel code over to the GEvent API was rather simple – what was more complex was attempting to fix a rather serious (in my opinion) usability issue with the mouse wheel zooming. My first intuition, when using the wheel-zooming, was to position my mouse cursor over where I wanted to zoom to then flick the mouse wheel up. However, moving the mouse wheel up causes the map to zoom in into the current map position – completely ignoring the position of the mouse. I’ve added the code to correctly capture the current lat/long of the cursor and pass it into the GEvent.
However, one issue still arises: Zooming in to the point you had your mouse over re-positions that point to the center of the screen – not where your mouse is. This means that you then have to move your mouse back to the center of the screen to continue zooming in. To fix this, I’ve written a method that can be used to properly scale the points to where they should be – this will even help the ‘click zoom‘ code that I developed earlier this week. You can see this for yourself in this demo. In that demo I use the following code to capture the mouse wheel movements and zoom in correctly:
GEvent.addListener( map, "wheelup", function(p){ if ( map.getZoomLevel() > 0 ) { map.centerAndZoom( p.scaleRelative( map.getCenterLatLng() ), map.getZoomLevel() - 1 ); } }); GEvent.addListener( map, "wheeldown", function(p){ if ( map.getZoomLevel() <= 16 ) map.centerAndZoom( p.scaleRelative( map.getCenterLatLng(), -1 ), map.getZoomLevel() + 1 ); });
The next feature is purely fun: Map in Map Support. Similar, in concept, to having ‘Window in Window’ on a TV screen, this displays a small, zoomed out, map in the corner of your main map.
Really, a demo would probably best illustrate this concept.
This was a test, for me, to see how easy/hard it would be to 1) Embed HTML on top of a map and 2) Put a Map in that HTML. The answer to both is: Easy and Very Easy. It took a little bit of poking around in the API to try and figure out how to get the HTML onto the map (looking at the code for similar functions, such as GSmallMapControls, helped a lot) – but once that was solved, adding a Map on top was as easy as ‘new GMap()’.
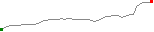
06/01 153 to 08/19 305
Now, a question to my readers (and if my recent analysis is correct, there’s over 300 of you): Did you enjoy this week long feature – looking at Google Maps? If you did/didn’t what specifically did you like/not care for? Any input would be greatly appreciated! Thanks in advance – I’m looking forward to do this again, very soon!
Matt Croydon (August 19, 2005 at 9:53 am)
John,
I’ve been reading your writing via RSS for a couple of months now, and yes I loved the Google maps feature.
–Matt
Jason (August 19, 2005 at 12:45 pm)
I’ve been enjoying most of the parts to the Google Maps tutorials. I’ve worked a bit with the Google Maps API so I know some of its shortcomings and it has been nice to see your improvements. I particularly love the scrollwheel zooming and have been waiting for today’s part since you first mentioned it.
Josh (August 19, 2005 at 2:11 pm)
Keep up the good work John. Sadly the project that I spend most of my time on would benefit greatly from some Gmaps, but it’s an internal firewalled app and hence doesn’t satisfy Google’s TOS. But what you’re doing is great and I’ll definitely keep reading (via Bloglines).
Any feedback from the Google guys on the series? I would very much like to see scroll wheel zooming implemented on the main site.
John Resig (August 19, 2005 at 3:54 pm)
Thanks a lot for the support – I really appreciate it!
Josh No feedback yet – I think I’m going to package everything that I wrote together into one big extension and then announce it on the Google Maps API mailing list. Itd even like to write up some proper documentation (like what is available on the API web site). Maybe I’ll pull this all together this weekend and release it, time permitting.
Jordan Sissel (August 19, 2005 at 5:07 pm)
Despite my having no intentions of using the google maps api any time soon, you’re week-long segment was quite informative and was something to look forward to reading daily this week.
Very well done, Bundt Cake Johnny ;)
Jason (August 23, 2005 at 10:37 am)
John,
Awesome tutorial series, well written and very creative. Keep up the good work!
Jason
John Resig (August 23, 2005 at 11:38 am)
Jason Thanks a lot – I really appreciate it!
Steve (August 24, 2005 at 9:35 pm)
Great series! I would love to see more! Can’t wait for the final code and documentation.
Steve
Tony (September 1, 2005 at 1:06 am)
Thank you for the impressive display of talent and ingenuity. I look forward to future suprises.
Cheston (September 1, 2005 at 4:37 pm)
Very interesting stuff, hopefully I will be able to find the time to actually play with google maps api in the near future, good work John.
The master would approve. ;)
Hiro Protagonist (September 1, 2005 at 4:59 pm)
Great Stuff, John!
Unfortunately, the mouse wheel zooming is not working properly for me in Firefox. It kinda works in certain situations (simple maps), but when I add layers on top of that map it now longer does.
Any ideas?
Hiro Protagonist (September 1, 2005 at 7:14 pm)
I just discovered that it only breaks under Linux, it works fine on Firefox on my Windows box. Strange :(
Ian (September 2, 2005 at 1:59 am)
John, I am in the exploratory stages of implementing the Google Maps API on a bushwalking site. Your very informative and helpful postings have given me inspiration for some of the features that I would like to build into our application. I particularly liked the demo of the map-in-map and will look for the fuller discussion on your site. Great stuff and thank you for generously sharing your creativity.
Piksar (September 14, 2005 at 10:16 pm)
This is actually among the best google maps API resource on the net. Almost everything is covered. Kudos to the author. What I’m looking forward is for you to tackle the map directions(from here to here) that is found on the maps at google site. I dont think it was documented in their API. I would love to see that form inside the balloon.
Thanks and Regards,
piksar
Bernie Connors (September 16, 2005 at 1:42 pm)
John,
This is a fabulous resource for all of us who are experimenting with the Google Maps API. I hope you continue to share the functions and code snippets that you write. At first I thought the Google Maps API would be beyond my skills but using the examples provided by yourself and others I have managed to embed some Google maps into my website.
Thanks,
Bernie Connors, P. Eng.
GIS Manager
Bernie Connors (September 16, 2005 at 1:50 pm)
RE: Map in Map
Would it be possible to keep the scale of the small map constant and just vary the size of the box? That is how this type of feature is usually implemented in a GIS.
Bernie Connors, P. Eng
GIS Manager
Bernie Connors (September 16, 2005 at 1:58 pm)
Here is an idea you might find interseting:
Is it possible to prevent the user from panning or zooming beyond a certain area? For example, I would like to set a default map view and zoom level that would present a map of the province of New Brunswick, Canada. And I want to prevent my user from:
1. zooming out to a larger view or
2. panning beyond the original view
Maybe you could call it a GFence?
Why would I want to do this? Because the overlay data I want to present is only pertinent to New Brunswick.
Bernie Connors, P.Eng
Gis Manager
Christophe (September 26, 2005 at 7:19 am)
We’re using Google Maps to plot scuba dive sites into maps. GM helps! Javascript functions to add layers are very quick to add. Maybe we’ll have a complete GIS solution in the future.
Chris
Wannadive.net team
Bruce Prochnau (October 1, 2005 at 3:17 am)
The series is awesome and extremely helpful. Very well done. As a webdeveloper good programmers are really appreciated.
Unfortunately, perhaps docs are still not available? On gaddress.
Internet explorer gives an error and firefox says something wrong with script prevents it from working.
Yet it works great on your site!
So I must be lacking something on server. Dang, this is the script I have searched the world fo and need most of all. Any feedback appreciated, desperately.
Thank you for all your work, awesome stuff.
noiv (December 26, 2005 at 12:04 pm)
I’ve found that map.getZoomLevel() tends to return a string,
so map.getZoomLevel() + 1 leads to unwanted results.
parseInt has helped then.
Anycase useful tutorial!
ROhan Kini (March 5, 2006 at 5:50 am)
Awesome sessions. Would love to see more of these.
Nice concept :-)
alfred (June 18, 2006 at 1:16 pm)
I dont think you know about new brunswick road maps. are you or not?
alfred (June 18, 2006 at 1:19 pm)
DO YOU KNOW ABOUT NEW BRUNSWICK ROAD MAP@
alfred (June 18, 2006 at 1:20 pm)
DONT KNOW FUCK ALL/
Immad (November 30, 2006 at 6:24 pm)
Unfortunately this didnt work for me in Firefox not sure why. The following works but it doesnt do the nice centering. There must be a way to combine the two techniques I will look into it at some point:
26 GMap2.prototype.wheelZoom = function(event)
27 { if((event.detail || -event.wheelDelta)
Immad (November 30, 2006 at 6:25 pm)
Hmm, cuts it off, anywa its on http://groups.google.co.uk/group/Google-Maps-API/msg/22adcdbf42cea0f9?dmode=source
Norbert (December 22, 2006 at 5:10 am)
hm, it’s realy not working on any of my browsers:?
icandothat (August 30, 2007 at 6:29 pm)
Doesn’t work for me either. Did something break in your demo? I’m using IE 6 and FF 2.0.0.6
Wheeling for zoom is something I’d like to do.