Google Chrome has taken the browser world by storm. It makes for an exciting release, no doubt, especially with a brand new JavaScript engine on the table.
However the most important question, to JavaScript developers, is: How will this affect my normal development routine? I’ve done some research, talked to some developers, submitted some bugs and come back with a list of things that you should probably be aware.
In all, there are some exciting tweaks, simple bugs, and baffling removals.
Timer Delay
Absolutely the most exciting change: Timer delays are the value that you set. For example if you do:
setTimeout(someFunction, 2);
then someFunction will attempt to execute in 2 milliseconds (depending on if there’s any other extenuating circumstances, naturally).
The only exception to the above is if a timer delay of < 1ms is provided then it will be rounded up to 1ms. I chatted with Mike Belshe, the developer who implemented timers in Chrome, and he hopes to completely remove that delay at some point. In browser development every millisecond counts.
I’ve done some analysis on this in the past and found that all browsers bottom-out at a certain point. Generally it’s around 10-15ms – if you provide a timer delay any smaller than that value it’ll be rounded up to the browser’s minimum.
There is no specification stating the level at which a timer minimum should work – and that’s a good thing – think of a mobile device. If a processor-limited mobile device wanted to raise the minimum bound for a timer it should be allowed to do so. At the same time a browser should be able to provide any lower bound that it can.
The obvious question becomes: Why aren’t other browsers doing this? There are a couple reasons:
- It will allow the user to peg the CPU, blocking the browser’s user interface. This isn’t an issue in Chrome since each site is contained within its own process (the CPU will still be pegged but only the host site will be affected).
- It may have some unforeseen side effects. This is the tricky part that no one is sure about at this point. It may cause weird problems in some sites – but no one is really sure yet. Chrome is being the canary-in-the-coalmine on this, keeping track of any new problems.
One muted question existed concerning performance tests. If a performance test used a timer interval less than 10ms would Chrome get an unnecessary advantage? The Chrome team did some research and they were unable to find any tests that did this – but I’m sure if one such test existed it could be, easily, rewritten to work better across browsers.
No Script Execution Dialog
In most other browsers if you try and run a consecutive piece of JavaScript for too long (for most browsers it’s around 5 seconds) a dialog will pop up asking the user if they wish to stop the execution of script on the page.
No such dialog exists in Chrome. Since script execution is confined to each process they simply allow it to run unchecked. If you wish to stop execution you must close the tab and re-open it. I’m not sure if this is the right UI for the task (maybe if a long-running script is executing and the user closes the tab then a dialog should pop up).
Personally, I’ve been having fun with this – keeping a page with a while(true){}
open at all times, while running Chrome.
Update: A number of readers have reported that they actually have been able to trigger a long-running script error dialog. Although there doesn’t seem to be much rhyme-or-reason as to when it appears. (I wasn’t able to get it to come up, for example.) Here’s a picture from Andrew Hawken:
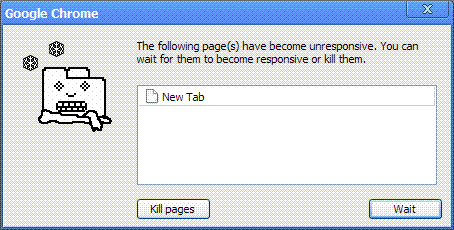
for loop order
Currently all major browsers loop over the properties of an object in the order in which they were defined. Chrome does this as well, except for a couple cases (V8 bug, Chrome bug).
You can see the issue in the following demo:
var obj = {first: [1, 2, 3], second: "blah"}, str = "<b>A property contains an array:</b><br/>", count = 1; for ( var i in obj ) { str += (count++) + ": " + i + "<br/>"; } str += "<hr/><b>Properties all contain strings:</b><br/>"; obj = {first: "blah", second: "blah"}; count = 1; for ( var i in obj ) { str += (count++) + ": " + i + "<br/>"; } window.onload = function(){ document.body.innerHTML = str; };
If an object contains a value which is not a primitive (as is the case in the first example) then its properties will be enumerated in a different order from which they were defined.
This is an interesting bug due to one fact: This behavior is explicitly left undefined by the ECMAScript specification. In ECMA-262, section 12.6.4:
The mechanics of enumerating the properties … is implementation dependent.
However, specification is quite different from implementation. All modern implementations of ECMAScript iterate through object properties in the order in which they were defined. Because of this the Chrome team has deemed this to be a bug and will be fixing it.
Text Node Serialization
This is, currently, causing the only regression in jQuery, for Chrome. It’s an odd WebKit bug which causes < and > characters to not be serialized to < and > when put in a text node. This has already been fixed in WebKit trunk and will be fixed the next time Chrome syncs its source.
Gears / Client-Side Storage
This is a tough nut to crack: Google has opted to bundle Gears directly with Chrome (which is a whole other discussion) but has explicitly removed WebKit’s HTML 5-compatible Client-Side Storage API.
Gears has its SQL database API bundled (contained within its own namespace and using its own API – different from the HTML 5 API) which makes this especially strange. Why, when there’s two client-side storage implementations on the table, would you pick the one that’s less spec-compliant?
I’m willing to give them the benefit of a doubt, especially considering that the Chrome FAQ mentions this case explicitly, but it definitely leaves me feeling quite confused.
Honestly, not much has changed in Chrome, over WebKit/Safari 3.1. The Chrome team hasn’t written any new features (save for those contained within Gears) or implemented any new specifications (quite different from the JavaScript situation in Internet Explorer 8). I realize that we’re still very early in the release of this browser and I hope to see many excellent contributions make their way into the Open Source WebKit codebase via the Chrome team.
Steve Streza (September 5, 2008 at 6:38 pm)
I have been quite pleasantly surprised at how complete V8 is. It was really nice loading up my company’s web site (powered by jQuery, of course) and testing all of my code and seeing it all just work. And it’s a testament to the jQuery team that there’s only been one regression. Ensuring application code works is vastly different from ensuring library code works.
Robert Accettura (September 5, 2008 at 7:24 pm)
Ditto on the Gears vs HTML 5 API. I said a few months ago I was concerned with the fragmentation here. I don’t understand why they didn’t disable the relevant Gears code instead pushing people to HTML 5 (or better yet, update Gears appropriately).
While they may have good intent to support it in a future release, during this time in between, developers are left with a choice… which favors the non-standard Gears implementation since it can be installed in more places. When business makes the decision… standards takes a back seat, something I don’t like to see.
Iraê (September 5, 2008 at 8:04 pm)
I am not really bothered by Google’s decision not to support HTML5 API in their first release for some reasons.
First they are building a browser from scratch, they will have an enormous work anyway to support the current features the other players support already. Why to spend time supporting features that an majority of developers won’t use because IE6 and IE7 will last for a long time yet?
Secondly, if the Gears team is planing to support the HTML5 spec and the Chrome will always ship it bundled, why can’t they choose to wait their implementation over to using Webkit right now?
And lastly, some non-standard feature implementations in the past wore not adopted and others non-standard are used today across all browsers (i.e. innerHTML in Javascript). Let’s await and see if the Gears implementation become popular, may be we could see some changes in the spec, who knows?
And of course, by their movement we can see an growing numbers of developers deploying features using gears because of chrome. They are giving a little push to their Gears comrades for sure.
Maciej Stachowiak (September 5, 2008 at 8:17 pm)
@Iraê It’s not that they didn’t implement a particular new feature that they’d have to write “from scratch”. The third-party engine they used when building their browser “from scratch” already had HTML5 support, which they turned off. Instead they chose to ship a Google extension providing a competing API, which they had to adapt to their version of an engine.
I do think they will provide the standard APIs in time, but I think this shows that they prioritized their proprietary APIs over open standards.
John Resig (September 5, 2008 at 8:18 pm)
@Iraê: I disagree completely. “First they are building a browser from scratch” that’s not true at all. This feature was already done for them – it came as part of the WebKit engine! They had to forcefully go through and remove it from the source (extra work) just to get to where they are. It required more work, on their end, to do this than just leaving it in.
“Why to spend time… because IE6 and IE7 will last for a long time yet?” This doesn’t make any sense. Why bother implementing any new standard if IE6 and 7 will never support them.
“Secondly, if the Gears team is planing to support the HTML5 spec and the Chrome will always ship it bundled, why can’t they choose to wait their implementation over to using Webkit right now?” Because right now their implementation doesn’t match the specification. It matches their own API. They had an implementation that completely worked – it was already there, baked in to the engine.
Since they actively removed the working API developers have no choice but to try and use the Gears API. Removing this runs completely contrary to their stated goals:
http://gearsblog.blogspot.com/2008/04/gears-and-standards.html
“Let’s await and see if the Gears implementation become popular, may be we could see some changes in the spec, who knows?” Because it’s not going to happen? Unless the W3C decides to add a new “gears” global object and place all pieces of HTML 5 inside of it, it’s just not going to come around.
“They are giving a little push to their Gears comrades for sure.” Shouldn’t they be pushing their “standards comrades” first – Gears second?
gerard (September 5, 2008 at 8:37 pm)
I stopped reading at “no support for HTML 5”.
John Resig (September 5, 2008 at 8:56 pm)
@gerard: Huh?
Daniel Luz (September 5, 2008 at 10:18 pm)
@gerard: I guess that means you stopped reading at your own comment? Nice meta-reference!
Samer Ziadeh (September 5, 2008 at 10:43 pm)
I think Chrome did ask me if I wanted to abort execution when I did a while(true)
Aristotle Pagaltzis (September 5, 2008 at 10:45 pm)
[Editorial note: the bare left-angle-bracket you used sort of breaks the post in some readers; in mine, the line reads “The only exception to the above is if a timer delay of Mike Belshe, the developer who implemented timers in Chrome” – which is funny, but probably not intended.]
John Resig (September 5, 2008 at 11:05 pm)
@Samer Ziadeh: Do you have some proof? Considering how long my while(true) page has been running, for me, I somehow doubt that this is the case.
@Aristotle Pagaltzis: Ah thanks, fixed.
Maciej Stachowiak (September 5, 2008 at 11:50 pm)
By the way, one correction. It is a little inaccurate to say that the Gears guys did extra work to disable WebKit’s database support. The work was probably limited to turning off an ifdef, and truly supporting it would have required making the back end work with their process architecture would have required nontrivial work (which they now plan to do).
But I do think it is fair and correct to say that they prioritized the (likely lesser but still nonzero) work to support Gears over the work to support standards. Indeed Gears itself has not done much to move towards the HTML5 standards APIs, which, to their credit, they have been a big part of designing.
The truth is nuanced here. Google isn’t being nasty, but their actions so far do not lend substance to their stated support for standards.
Dipen (September 5, 2008 at 11:51 pm)
is it possible google chose to go with gears implementation for client side storage rather than web kit implementation of HTML5 because of android? They want app’s ready to run on android?
Could that be a reason?
Nosredna (September 6, 2008 at 12:16 am)
…for most browsers it’s around 5 seconds…
If I recall correctly, that’s changed. I think it’s more like 15 seconds or more in the latest browsers. I wish I would have kept my notes on the the last time I was running into the problem.
bob (September 6, 2008 at 12:33 am)
Here’s a screenshot of Chrome’s warning if a script is running unchecked. For me this happens around the one minute mark.
It’s in dutch, but I’m sure you’ll get what it says:
http://webtwee.net/2008-09/aed94c99e8d3781ebb4f176547d1367d.png
Iraê (September 6, 2008 at 12:49 am)
@John Resig Some of the thing I said wore a little misunderstood (may be a problem with my English), some I was completely wrong indeed, and for that I’m sorry.
By “from scratch” I meant everything else, like UI, separate processes, sandboxes, and specially V8.
In my point of view they should bring the standards first and secondly gears. I agree with you. But I’m still not convinced this is the best choice for them.
Once you said yourself, when talking about the jQuery plugin architecture (correct me if I’m wrong) that every new feature was not a meter of time to code and usefulness to the lib user. Every feature is something to maintain, debug, optimize, test and etc.
By disabling the SQL API they didn’t have to look into performance, sendboxes, and many more issues. IMHO this was probably a decision of cost/release date and the time spent to remove it was probably smaller than maintaining it in the first beta.
As Maciej Stachowiak said: they could wish to make de SQL another process so the user can kill it and in this case the UI and host page will have to handle the error flawlessly. If this is so, they will have to patch the SQL API code a lot.
Or even, may they be expecting to make some kind of wrapper/translator to use gears to supply the SQL the HTML5 way? I don’t know, Is this is technically possible?
Adhip Gupta (September 6, 2008 at 4:14 am)
while(true) {}
did show me a warning… in about ten seconds or less!Lucho (September 6, 2008 at 4:46 am)
From developer build revision 1785,
“Try again to begin compiling the files that implemen the HTML5 database API. This time, I added a project dependency from WebCore to sqlite on every solution that includes WebCore.Review URL: http://codereview.chromium.org/466”
Is now HTML5 database API enabled?
Malte (September 6, 2008 at 5:20 am)
Its actually quite easy to use JS to build an API that looks like the HTML5 db api on top of the Gears DB (Although it won’t be truly asynchronous).
I implemented such a wrapper API and than put an OR-Mapper on top that only uses the HTML5 API and it works quite far so far.
http://code.google.com/p/joose-js/wiki/JooseOnGears#Database
I think it was a mistake to only offer an asynchronous DB API. Although it might be good for page responsiveness, most queries in a client scenario are ultra fast and DB development is way easier in a synchronous environment.
Andrew Hawken (September 6, 2008 at 5:50 am)
Chrome *does* display an unresponsive dialogue.
http://exhale.co.uk/tmp/chrome.gif
running a web page with a while(true){} produces it, as does a
javascript:while(true){}
in the URL/search box, but only in some circumstances. I can force it to happen if its the first thing I do in the first tab after opening Chrome, however I have no idea what the rules actually are.Andrew Hawken (September 6, 2008 at 7:07 am)
Thinking some more : I wonder if its something to do with % of total resource or resource used by the browser. I’m running it on a fairly old (and overloaded; it needs a reinstall) laptop. If I get a chance I’ll give it a try on my decent desktop.
John Resig (September 6, 2008 at 7:25 am)
@Dipen: It’s not clear what their reasoning is – we’ll have to ask them, I guess.
@Nosredna: It’s definitely still quite short. I hit it all the time in the performance testing that I do.
@bob, Adhip, Andrew: Thanks for the confirmation, I’ve updated the blog post. Now we just need to figure out what triggers it! I was able to have the while(true){} running in my browser, consuming 100% of the CPU, and not have any dialog appear so I’m not sure what the trick is.
@Iraê: “By disabling the SQL API they didn’t have to look into performance, sandboxes, and many more issues. IMHO this was probably a decision of cost/release date and the time spent to remove it was probably smaller than maintaining it in the first beta.”
I can agree that their motives may have been pure (don’t add it to the release in favor of a simpler overhead) but their actions speak louder than their words. Whatever issues they had with having the SQL API be accessible from a process were fixed in order to make Gears work, but not fixed in order to get the standards-compliant functionality to work. It’s really a matter of prioritization.
@Lucho: Definitely doesn’t look like it’s enabled, only that they’re starting to review the code for addition to the browser.
@Malte: Interesting points, thanks for bringing it up;
Slim Amamou (September 6, 2008 at 8:24 am)
Actually i think the major innovation introduced by chrome lies in the process. specifically large scale automated user tests (http://slim.soup.io/post/4982833/via-Google-Chrome).
beside the technical challenge, i think this introduces a major innovation in OpenSource governance. if you own the tests, you own the project.
Dave Largo (September 6, 2008 at 9:23 am)
“It will allow the user to peg the CPU…”
Could you please explain the meaning of this? Does it mean to hog the CPU or something like that?
suntzu (September 6, 2008 at 9:37 am)
Has anybody tried processingjs library on the chrome?
PDF reading is also a big issue in Chrome currently. It hangs the browser (cpu surge to 80-100%)
John Resig (September 6, 2008 at 10:03 am)
@Dave Largo: Yeah, it would be able to hog the whole CPU much in the way a while(true) loop could (especially limiting the availability of the user interface, making it hard to click).
@suntzu: Not yet, but I’m willing to bet that it’s as smooth as butter. If there’s anything that this change will provide, in Chrome, is super-smooth animations.
Sengan Baring-Gould (September 6, 2008 at 11:06 am)
Go to the web inspector’s console. Type while (true) {};
The alert will come up after a few seconds. You may lose some other pages too (one or more tabs per process).
John Resig (September 6, 2008 at 11:22 am)
@Sengan Baring-Gould: Go to this page in Chrome and see if the dialog pops up for you.
Nosredna (September 6, 2008 at 11:32 am)
>>@Nosredna: It’s definitely still quite short. I hit it all the time in the performance testing that I do.
I don’t think so. I just tried in Firebug and with your bug page and Firefox3.01 goes and goes and goes without throwing the unresponsive script dialog.
Possibly there’s a difference between Mac and PC? I’m running in XP, and Firefox shows no hint of throwing he alert.
Malte (September 6, 2008 at 11:51 am)
Just for the record: Here is the source that implements the HTML5 wrapper API for the gears database:
http://code.google.com/p/joose-js/source/browse/trunk/examples/simple_orm/async/ORM.js#5
John Resig (September 6, 2008 at 12:02 pm)
@Nosredna: It sounds like you’ve opened about:config and have configured ‘dom.max_script_run_time’. The default is 10 seconds in Firefox 3, for what it’s worth.
Nosredna (September 6, 2008 at 12:28 pm)
@johnresig
Haha! Yeah, I probably did that. I just set it back to default.
Nosredna (September 6, 2008 at 12:28 pm)
I KNEW that it got longer. So it’s 10 seconds for FF3.
Ivan (September 6, 2008 at 6:54 pm)
I think the ecma specification definitely needs to have the property order fixed. This is something I (and I’m sure other developers) depend on for behavior. otherwise, a simple configurable json object now needs ot be wrapped in an array in order to have predictable results…
i’d say chrome should go with the flow in this case and someone get this back to the spec team!
andy (September 7, 2008 at 12:54 am)
ive been comparing the performance of a couple of apps between the nightly build of firefox with JIT enabled and chrome.
this game:
http://www.schillmania.com/arkanoid/arkanoid.html#arcade
runs much faster and without any jumpiness in chrome than in minefield
as does a few processing.js examples i’ve tried.
What are the likely reasons for this disparity?
Chris (September 7, 2008 at 4:27 pm)
I like the way that Chrome handles view source, opening a new tab with line numbers and hyperlinks. It raises the question, “what would be the ultimate view source”? And the answer is probably Firebug.
So how about replacing Firefox’s View Source with Firebug? Press Ctrl-U to examine source, even change it and debug it, all in a new tab?
Markus.Staab (September 8, 2008 at 8:06 am)
@John:
you can have a look at the hang-dialog, if you type about:hang in the omnibox.
mo (September 8, 2008 at 10:02 am)
I’d like to think Chrome is good but afraid my web app that loads an xml doc won’t work any more.
The code (that works fine in FF and IE) looks like this:
if(document.implementation && document.implementation.createDocument)
var myDoc = document.implementation.createDocument("","",null);
else
var myDoc = new ActiveXObject("MSXML2.DOMDocument");
myDoc.async = false;
myDoc.load(someURL);
Of course this is inside a try/catch block and I get an exception when the document loading part is reached. the exception goes like this:
# has no method ‘load’ error.
Any help on this will be much appreciated. I have already checked Google Forums and found a similar post but without any reply.
Breton (September 8, 2008 at 6:55 pm)
@mo: It’s because ActiveX is a proprietary microsoft technology, not a standard. You should only expect that code to work in IE. I have no idea why it works in firefox (mozilla is probably just being nice somehow).
Breton (September 8, 2008 at 11:02 pm)
Doh I should have looked at your code more carefully.
Sorry if I implied anything impolite.
Edin (September 9, 2008 at 3:50 am)
I’m wondering (probably as all you do) how is it possible that instead of cooperation between existing browsers to merge they engines into one standard html/css rendering we now got brand new browser with it’s own standard. I’m not speaking about javascript only, there is problem with layout of some of my web-pages. They were all working great in all browsers (IE, FF, Opera… ) and now with Chrome I’ve got some divs lost all over screen (unwanted)
:(
mo (September 9, 2008 at 5:20 am)
Hi Breton, no worries. I was in a rush and left a poorly formatted bit of code.
Edin, consider yourself lucky. My application fails during initialisation (while reading it’s config data) so I haven’t been as far as looking at the layout.
anyway, here’s some further info on this:
http://groups.google.com/group/v8-users/browse_thread/thread/12a7d110a558e789
Dave (September 10, 2008 at 12:00 am)
A timer resolution under 1ms is not feasible on Windows. Win32 timers have a practical resolution of about 20ms. The multimedia timers may provide slightly better resolution, but it’s hard to get under 10-12ms.
Interrupt servicing and queued DPCs, as well as thread scheduling, mean that you simply can’t be guaranteed the kind of precision you’re after on NT-based operating systems.
thanwa (September 10, 2008 at 1:23 am)
Dear Mo,
I accidentally stumble upon this page when I find if anyone in the Internet has the same problem as mine to see if it was my poorly coding or else…
I had the same problem as yours.
I found the Implementation object in Chrome doesn’t work as the same as other Firefox/Mozilla/Seamonkey counterpart. (I would not prefer it’s a bug but I don’t know what has been changed for Chrome’s Implementation object)
I, instead change the code to use XMLHttpRequest object instead.
Try this code then,
function loadxml(xmlfile){
var xmldoc
if(window.XMLHttpRequest){
xmldoc = new window.XMLHttpRequest();
xmldoc.open("GET",xmlfile,false)
xmldoc.send("")
return xmldoc.responseXML;
}
else if(window.ActiveXObject){
xmldoc = new ActiveXObject("Microsoft.XMLDOM");
xmldoc.async = false;
xmldoc.load(xmlfile)
return xmldoc
} else{
alert("XML loading not supported.");
return null;
}
}
mo (September 10, 2008 at 6:25 am)
Hi Thanwa
Thanks so much. Just tried your example and it worked beautifully in all three browsers. Think using XMLHttpRequest is a better approach anyway as this is the core of AJAX which is expected to be better supported by the ‘good’ browsers:-)
By the way I thought Chrome ran my application quite well. Didn’t see any issues in the CSS handling either.
best regards
mo
mauk (September 12, 2008 at 7:16 am)
A remark about for loop order:
“all major browsers loop over the properties of an object in the order in which they were defined”. But not in Rhino:
for (i in { a:1, b:2, aa:3, c:4 }) print(i);
prints c,aa,a,b
Mauk
sohbet (September 30, 2008 at 9:02 am)
I think chrome ll be a rival of firefox in future. But if didn’t be, i was be happy more. Because we’r trying to make our sites compatible with IE, Mozilla, Opera, Safari; thats enough brotha. I don’t want to try to compatible my sites for Chrome.
Look, http://www.nsohbet.com/ i did this site in 4 days. Site was simple, but i didn’t compatible it with all browsers.
Anyway, thanks for great article. I think i should add your blog to my feeds.
Kind regards,
Mehmet Tahta
Ken Ko (October 15, 2008 at 9:04 am)
@mauk: regarding loop order in rhino, from memory I think it is a rhino bug which is fixed in the nightly build
cris (November 2, 2008 at 1:04 pm)
i dont like the new google chrome browser, i found it unpractical maybe they will enhanced it but for now FF 2.0 is the best imo
sohbet (November 2, 2008 at 9:14 pm)
Oh, c’mon cris, firefox 3 is the best. also i changed my mind, before my last post, i said i like chrome, but now i don’t like. it’s not enough and i think it ll be never enough.
cris (November 7, 2008 at 9:28 am)
i agree with your chrome perspective, i found it’s interface like”oh yeah we’re on the comands of a starship”, regarding FF i havent updated to 3 , 2 it’s cool for me and i dont like those icons they added in the adress bar, keep thing simple.