I’ve created a simple tool for landing pull requests from Github, which I’m calling “Pulley“.
Landing a pull request from Github can be annoying. You can follow the instructions provided by Github (pulling the code, doing a merge) but that’ll result in a messy commit stream and external ticket trackers that don’t automatically close tickets. The series of commands that you run will typically look something like this:
git checkout -b branchname master git pull http://url/to/otherrepo branchname git checkout master git merge branchname git push origin master
Additionally you can pull the code and squash it down into a single commit, which lets you format the commit nicely (closing tickets on external trackers) – but it fails to properly close the pull request. The commands for which look something like this:
git checkout master git checkout -b bug1234 git pull http://url/to/otherrepo branchname git checkout master git merge --no-commit --squash bug1234 git commit -a --author="Original Author" # To get the author: git log | grep "Author" | head -1
Pulley is a tool that uses the best aspects of both techniques. Pull requests are pulled and merged into your project. The code is then squashed down into a single commit and nicely formatted with appropriate bug numbers and links. Finally the commit is pushed and the pull request is closed with a link to the commit.
Pulley is written using Node.js – thus you’ll need to make sure that you have Node installed prior to running it.
How to use:
Download a copy of Pulley or install it via npm: npm install pulley
and configure the repo and bug tracker details in the pulley.js file (this is only necessary if you use a custom, non-Github, issue tracker).
You’ll also need to set up your Github username and token into your Git repo. More details can be found in the Github docs.
Once that’s complete you can run the following command:
node pulley.js PID # Where PID is the Pull Request ID
For example running the command node pulley.js 332
on the jQuery repo yielded the following closed pull request, commit, and tickets:

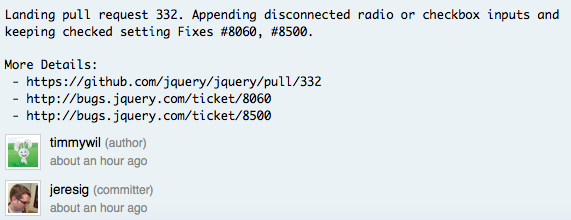
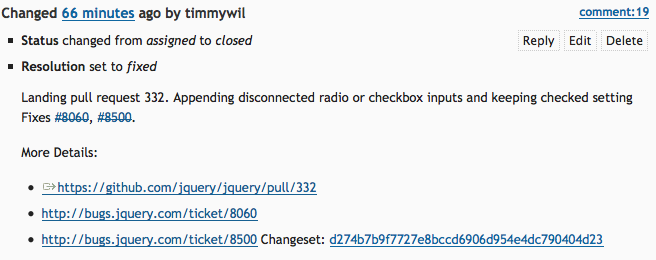
The configuration is still a bit janky but it works well enough for me – I’m looking forward to landing a few pull requests to improve it.
Dean (April 21, 2011 at 10:40 pm)
how about a npm package to make it easier for us npm users? :-)
Rick (April 21, 2011 at 10:43 pm)
I’d suggest setting up a git alias: `git config –global alias.pulley ‘!node pulley.js’`
Then you can run `git pulley `
John Resig (April 21, 2011 at 11:00 pm)
@Dean: Good call! It’s now up on npm:
http://search.npmjs.org/#/pulley
@Rick: Great tip, thanks!
Dustin Diaz (April 21, 2011 at 11:17 pm)
Super useful! Will grab a copy. You should make it an NPM package. so you can just type:
$ pully repo/123
So if you had multiple repo’s you can close out multiple tickets…
$ pull jquery/77
Dustin Diaz (April 21, 2011 at 11:27 pm)
FYI, what I was talking about was slightly different than having it just as an npm package. What I was getting at was to make a bin interface
https://github.com/isaacs/npm/blob/master/doc/json.md – see “bin”
John Resig (April 21, 2011 at 11:36 pm)
@Dustin: Excellent point! I just updated the npm package (v0.1.2 now) to have a proper bin that installs the “pulley” command. Thanks for the suggestion!
Alexander Beletsky (April 21, 2011 at 11:39 pm)
Wow, this is exactly something I’ve been looking for some weeks ago :)
Now, it is one more “push” to start with node.js :)
Dustin Diaz (April 21, 2011 at 11:48 pm)
@John, excellent. Totally using this.
Elijah Insua (April 22, 2011 at 1:02 pm)
@John, very nice utility! It sort of goes against my workflow though, as I like to run the new code against unit tests before pushing it to github
Mark Essel (April 22, 2011 at 1:34 pm)
@John, dig the tool idea although with my limited merging I haven’t had many issues with git or github’s default (using mostly os x opendiff/FileMerge is no meld though, any opinion on kdiff3 I haven’t swapped it in yet).
I bumped into a small issue with the package install:
messels-MacBook-Air:~ messel$ npm install pulley
npm info it worked if it ends with ok
npm info using [email protected]
npm info using [email protected]
npm WARN not a valid range. Please see `npm help json` 0.4 || 0.5
npm WARN not a valid range. Please see `npm help json` 0.4 || 0.5
npm WARN not a valid range. Please see `npm help json` 0.4 || 0.5
npm WARN not a valid range. Please see `npm help json` 0.4 || 0.5
npm WARN Not supported on [email protected] pulley
npm WARN not a valid range. Please see `npm help json` 0.4 || 0.5
npm ERR! [email protected] not compatible with your version of node
npm ERR! Requires: [email protected] || 0.5
npm ERR! You have: [email protected]
npm not ok
messels-MacBook-Air:~ messel$
It mentions I need 0.4 or 0.5, while I have 0.4.0. Should be ok right? I haven’t done much with node beyond a few tutorials including a groovy coffee server with nodemon so I may have taken a wrong step. I’m using nvm as well to maintain different versions of node.
John Resig (April 22, 2011 at 2:52 pm)
@Mark Essel: I think you may need an updated version of npm for that to work? I just added that line in since I saw it in other package.json files – so I’ve just removed it, you should be fine now (let’s hope).
Kartik (April 23, 2011 at 12:38 am)
Awesome tool… Any chance I can get it as a debian package so that I can install it on my Ubuntu box…
DWF (April 23, 2011 at 2:44 pm)
Does this have a mode that allows for the commit & post-commit to happen in a 2nd step?
I want to pull/squash, run tests/specs, possibly tweak code on the branch, then push and close.
If not, then I guess it’s time to fork and submit a pull request…
John Resig (April 23, 2011 at 5:07 pm)
@DWF: Not yet – but it’s definitely something that I wanted. It’d be nice to have a default “do everything quickly and land it right away” and another that’s “be more deliberate and let me tweak/test things and possibly change the commit message.” If you’re itching to do a pull, go to town!
Ryan Sharp (April 25, 2011 at 12:05 pm)
Unfortunate timing. This was just made largely redundant for people using GitHub — https://github.com/blog/843-the-merge-button
Mathias Bynens (April 25, 2011 at 12:09 pm)
@Ryan Assuming you want to test patches (and run unit tests) before pulling them in, Pulley is still the better solution here.
Bobby Eickhoff (April 26, 2011 at 11:39 am)
I could be mistaken, but in both of the original examples I don’t think you need to create a local branch. You just pull directly into master using the same lone-ref syntax for the refspec (i.e. ref with no colon):
git checkout master
git pull http://url/to/otherrepo branchname
git push origin master
and
git checkout master
git pull –squash http://url/to/otherrepo branchname
git commit
git push origin master